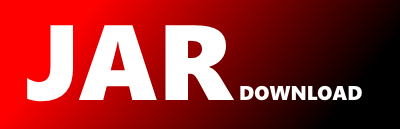
io.github.vmzakharov.ecdataframe.dataframe.DfJoin Maven / Gradle / Ivy
package io.github.vmzakharov.ecdataframe.dataframe;
import org.eclipse.collections.api.list.ListIterable;
import org.eclipse.collections.impl.factory.Lists;
final public class DfJoin
{
private DataFrame joinFrom;
private DataFrame joinTo;
private ListIterable selectFromJoined;
private ListIterable columnNameAliases;
private ListIterable
© 2015 - 2025 Weber Informatics LLC | Privacy Policy