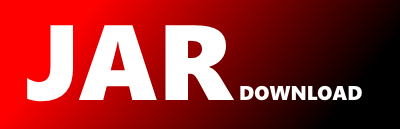
io.github.vmzakharov.ecdataframe.dsl.ProjectionExpr Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dataframe-ec Show documentation
Show all versions of dataframe-ec Show documentation
A tabular data structure based on the Eclipse Collections framework
package io.github.vmzakharov.ecdataframe.dsl;
import io.github.vmzakharov.ecdataframe.dsl.value.Value;
import io.github.vmzakharov.ecdataframe.dsl.visitor.ExpressionEvaluationVisitor;
import io.github.vmzakharov.ecdataframe.dsl.visitor.ExpressionVisitor;
import io.github.vmzakharov.ecdataframe.dsl.visitor.PrettyPrintVisitor;
import org.eclipse.collections.api.list.ListIterable;
import org.eclipse.collections.api.list.MutableList;
import org.eclipse.collections.impl.factory.Lists;
public class ProjectionExpr
implements Expression
{
private final Expression whereClause;
private final ListIterable projectionElements;
private final MutableList elementNames = Lists.mutable.of();
private final MutableList projectionExpressions = Lists.mutable.of();
public ProjectionExpr(ListIterable newProjectionElements)
{
this(newProjectionElements, null);
}
public ProjectionExpr(ListIterable newProjectionElements, Expression newWhereClause)
{
this.projectionElements = newProjectionElements;
this.whereClause = newWhereClause;
for (int i = 0; i < this.projectionElements.size(); i++)
{
Expression element = this.projectionElements.get(i);
if (element instanceof AliasExpr)
{
this.elementNames.add(((AliasExpr) element).getAlias());
this.projectionExpressions.add(((AliasExpr) element).getExpression());
}
else
{
this.elementNames.add(PrettyPrintVisitor.exprToString(element));
this.projectionExpressions.add(element);
}
}
}
@Override
public Value evaluate(ExpressionEvaluationVisitor visitor)
{
return visitor.visitProjectionExpr(this);
}
@Override
public void accept(ExpressionVisitor visitor)
{
visitor.visitProjectionExpr(this);
}
public Expression getWhereClause()
{
return this.whereClause;
}
public ListIterable getProjectionElements()
{
return this.projectionElements;
}
public ListIterable getProjectionExpressions()
{
return this.projectionExpressions;
}
public ListIterable getElementNames()
{
return this.elementNames;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy