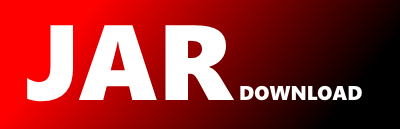
com.weloe.downloader.bufferPool.AbstractBufferPool Maven / Gradle / Ivy
package com.weloe.downloader.bufferPool;
import java.util.ArrayList;
import java.util.List;
import java.util.concurrent.BlockingQueue;
import java.util.concurrent.ScheduledExecutorService;
import java.util.concurrent.TimeUnit;
/**
* @author weloe
*/
public abstract class AbstractBufferPool implements BufferPool{
private final BlockingQueue logBuffer; // 日志项缓冲区
private final int flushTimeInterval; // 刷新时间间隔
private final ScheduledExecutorService executorService; // 定时任务执行器
public AbstractBufferPool(BlockingQueue logBuffer, int flushTimeInterval, ScheduledExecutorService executorService) {
this.logBuffer = logBuffer;
this.flushTimeInterval = flushTimeInterval;
this.executorService = executorService;
startFlushTask();
}
@Override
public final void put(T item) throws InterruptedException {
if (logBuffer.remainingCapacity() == 0) {
flush();
}
logBuffer.put(item);
}
protected void startFlushTask() {
executorService.scheduleWithFixedDelay(this::flush, flushTimeInterval, flushTimeInterval, TimeUnit.MILLISECONDS);
}
@Override
public final void flush() {
List items = new ArrayList<>();
// 取出缓冲区中的所有日志项
logBuffer.drainTo(items);
if (!items.isEmpty()) {
flushCallback(items);
}
}
@Override
public final void shutdown(){
executorService.shutdown();
flush();
}
protected abstract void flushCallback(List items);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy