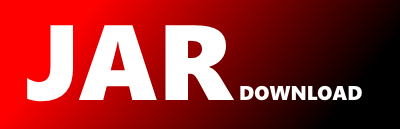
io.github.whyareyousoseriously.czcommonutils.util.BeanCopierUtil Maven / Gradle / Ivy
package io.github.whyareyousoseriously.czcommonutils.util;
import net.sf.cglib.beans.BeanCopier;
import java.io.*;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* @author chenzhen
* Created by chenzhen on 2019/12/25.
*/
public class BeanCopierUtil {
public static Map beanCopierMap = new HashMap();
public static void deepCopyObject(Object source, Object target) {
String beanKey = generateKey(source.getClass(), target.getClass());
BeanCopier copier = null;
if (!beanCopierMap.containsKey(beanKey)) {
copier = BeanCopier.create(source.getClass(), target.getClass(), false);
beanCopierMap.put(beanKey, copier);
} else {
copier = beanCopierMap.get(beanKey);
}
copier.copy(source, target, null);
}
public static List deepCopyList(List src) {
try {
ByteArrayOutputStream byteout = new ByteArrayOutputStream();
ObjectOutputStream out = new ObjectOutputStream(byteout);
out.writeObject(src);
ByteArrayInputStream bytein = new ByteArrayInputStream(byteout.toByteArray());
ObjectInputStream in = new ObjectInputStream(bytein);
@SuppressWarnings("unchecked")
List dest = (List) in.readObject();
return dest;
} catch (ClassNotFoundException | IOException e) {
e.printStackTrace();
return null;
}
}
private static String generateKey(Class> class1, Class> class2) {
return class1.toString() + class2.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy