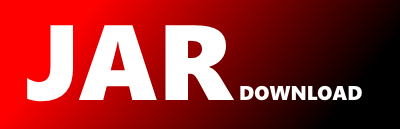
io.github.whyareyousoseriously.czcommonutils.util.ServiceResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cz-common-utils Show documentation
Show all versions of cz-common-utils Show documentation
Demo project for Spring Boot
package io.github.whyareyousoseriously.czcommonutils.util;
import com.alibaba.fastjson.JSONObject;
import io.github.whyareyousoseriously.czcommonutils.exception.ServiceResultException;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import java.io.Serializable;
/**
* service层返回对象列表封装
*
* @param
* @author chenzhen
*/
public class ServiceResult implements Serializable {
private Integer code;
private String message;
private T result;
private ServiceResult() {
}
public static ServiceResult success(T result) {
ServiceResult item = new ServiceResult();
item.result = result;
item.code = HttpStatus.OK.value();
item.message = "success";
return item;
}
public static ServiceResult success(int successCode, String message, T result) {
ServiceResult item = new ServiceResult();
item.result = result;
item.code = successCode;
item.message = message;
return item;
}
public static ServiceResult failure(int errorCode, String errorMessage) {
ServiceResult item = new ServiceResult();
item.code = errorCode;
item.message = errorMessage;
return item;
}
public static ServiceResult failure(int errorCode, String errorMessage, T result) {
ServiceResult item = new ServiceResult();
item.code = errorCode;
item.message = errorMessage;
item.result = result;
return item;
}
public static ServiceResult failure(int errorCode) {
ServiceResult item = new ServiceResult();
item.code = errorCode;
item.message = "failure";
return item;
}
public boolean hasResult() {
return result != null;
}
public T getResult() {
return result;
}
public Integer getCode() {
return code;
}
public String getMessage() {
return message;
}
public static void checkServiceResult(ServiceResult serviceResult) throws ServiceResultException {
if (serviceResult == null) {
throw new ServiceResultException("待检测的serviceResult内容为空");
} else {
if (serviceResult.getCode() != HttpStatus.OK.value()) {
throw new ServiceResultException(serviceResult.getMessage());
}
}
}
public static T dealwithResponseEntity(ResponseEntity> responseEntity) throws ServiceResultException {
if (responseEntity.getStatusCode().is2xxSuccessful()) {
ServiceResult body = responseEntity.getBody();
ServiceResult.checkServiceResult(body);
if (body.getCode() == HttpStatus.BAD_REQUEST.value()) {
throw new ServiceResultException(body.getResult().toString());
}
if (body.getResult()==null){
throw new ServiceResultException("请求下层返回结果为null");
}
return body.getResult();
} else {
throw new ServiceResultException(responseEntity.getStatusCode().getReasonPhrase());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy