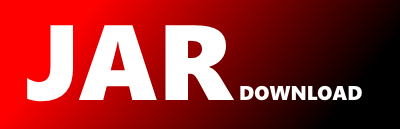
org.fiware.ngsi.model.NotificationVO Maven / Gradle / Ivy
package org.fiware.ngsi.model;
@jakarta.annotation.Generated("org.openapitools.codegen.languages.MicronautCodegen")
@io.micronaut.core.annotation.Introspected
public class NotificationVO {
public static final java.lang.String JSON_PROPERTY_AT_CONTEXT = "@context";
public static final java.lang.String JSON_PROPERTY_ID = "id";
public static final java.lang.String JSON_PROPERTY_TYPE = "type";
public static final java.lang.String JSON_PROPERTY_SUBSCRIPTION_ID = "subscriptionId";
public static final java.lang.String JSON_PROPERTY_NOTIFIED_AT = "notifiedAt";
public static final java.lang.String JSON_PROPERTY_DATA = "data";
@com.fasterxml.jackson.annotation.JsonProperty(JSON_PROPERTY_AT_CONTEXT)
@com.fasterxml.jackson.annotation.JsonInclude(com.fasterxml.jackson.annotation.JsonInclude.Include.NON_NULL)
private java.lang.Object atContext;
@com.fasterxml.jackson.annotation.JsonProperty(JSON_PROPERTY_ID)
@com.fasterxml.jackson.annotation.JsonInclude(com.fasterxml.jackson.annotation.JsonInclude.Include.ALWAYS)
private java.net.URI id;
/** NGSI-LD Name */
@com.fasterxml.jackson.annotation.JsonProperty(JSON_PROPERTY_TYPE)
@com.fasterxml.jackson.annotation.JsonInclude(com.fasterxml.jackson.annotation.JsonInclude.Include.ALWAYS)
private java.lang.String type;
@com.fasterxml.jackson.annotation.JsonProperty(JSON_PROPERTY_SUBSCRIPTION_ID)
@com.fasterxml.jackson.annotation.JsonInclude(com.fasterxml.jackson.annotation.JsonInclude.Include.ALWAYS)
private java.net.URI subscriptionId;
@com.fasterxml.jackson.annotation.JsonProperty(JSON_PROPERTY_NOTIFIED_AT)
@com.fasterxml.jackson.annotation.JsonInclude(com.fasterxml.jackson.annotation.JsonInclude.Include.ALWAYS)
private java.time.Instant notifiedAt;
@com.fasterxml.jackson.annotation.JsonProperty(JSON_PROPERTY_DATA)
@com.fasterxml.jackson.annotation.JsonInclude(com.fasterxml.jackson.annotation.JsonInclude.Include.ALWAYS)
private java.util.List data = new java.util.ArrayList<>();
// methods
@Override
public boolean equals(Object object) {
if (object == this) {
return true;
}
if (object == null || getClass() != object.getClass()) {
return false;
}
NotificationVO other = (NotificationVO) object;
return java.util.Objects.equals(atContext, other.atContext)
&& java.util.Objects.equals(id, other.id)
&& java.util.Objects.equals(type, other.type)
&& java.util.Objects.equals(subscriptionId, other.subscriptionId)
&& java.util.Objects.equals(notifiedAt, other.notifiedAt)
&& java.util.Objects.equals(data, other.data);
}
@Override
public int hashCode() {
return java.util.Objects.hash(atContext, id, type, subscriptionId, notifiedAt, data);
}
@Override
public java.lang.String toString() {
return new java.lang.StringBuilder()
.append("NotificationVO[")
.append("atContext=").append(atContext).append(",")
.append("id=").append(id).append(",")
.append("type=").append(type).append(",")
.append("subscriptionId=").append(subscriptionId).append(",")
.append("notifiedAt=").append(notifiedAt).append(",")
.append("data=").append(data)
.append("]")
.toString();
}
// fluent
public NotificationVO atContext(java.lang.Object newAtContext) {
this.atContext = newAtContext;
return this;
}
public NotificationVO id(java.net.URI newId) {
this.id = newId;
return this;
}
public NotificationVO type(java.lang.String newType) {
this.type = newType;
return this;
}
public NotificationVO subscriptionId(java.net.URI newSubscriptionId) {
this.subscriptionId = newSubscriptionId;
return this;
}
public NotificationVO notifiedAt(java.time.Instant newNotifiedAt) {
this.notifiedAt = newNotifiedAt;
return this;
}
public NotificationVO data(java.util.List newData) {
this.data = newData;
return this;
}
public NotificationVO addDataItem(EntityVO dataItem) {
if (this.data == null) {
this.data = new java.util.ArrayList<>();
}
this.data.add(dataItem);
return this;
}
public NotificationVO removeDataItem(EntityVO dataItem) {
if (this.data != null) {
this.data.remove(dataItem);
}
return this;
}
// getter/setter
public java.lang.Object getAtContext() {
return atContext;
}
public void setAtContext(java.lang.Object newAtContext) {
this.atContext = newAtContext;
}
public java.net.URI getId() {
return id;
}
public void setId(java.net.URI newId) {
this.id = newId;
}
public java.lang.String getType() {
return type;
}
public void setType(java.lang.String newType) {
this.type = newType;
}
public java.net.URI getSubscriptionId() {
return subscriptionId;
}
public void setSubscriptionId(java.net.URI newSubscriptionId) {
this.subscriptionId = newSubscriptionId;
}
public java.time.Instant getNotifiedAt() {
return notifiedAt;
}
public void setNotifiedAt(java.time.Instant newNotifiedAt) {
this.notifiedAt = newNotifiedAt;
}
public java.util.List getData() {
return data;
}
public void setData(java.util.List newData) {
this.data = newData;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy