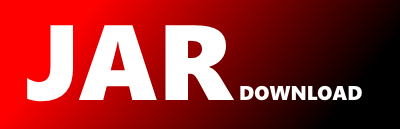
io.github.wj0410.cloudbox.tools.result.R Maven / Gradle / Ivy
The newest version!
package io.github.wj0410.cloudbox.tools.result;
import io.github.wj0410.cloudbox.tools.constant.Constants;
import org.springframework.lang.Nullable;
import org.springframework.util.ObjectUtils;
import java.io.Serializable;
import java.util.Optional;
/**
* // @JsonInclude(JsonInclude.Include.NON_NULL)
*
* @author wangjie
* @version 1.0
* date 2021年08月03日09时29分
*/
public class R implements Serializable {
private static final long serialVersionUID = 1L;
/**
* 成功
*/
public static final int SUCCESS = Constants.SUCCESS;
/**
* 失败
*/
public static final int FAIL = Constants.FAIL;
private int code;
private boolean success;
private T data;
private String msg;
private R(IResultCode iResultCode) {
this(iResultCode.getCode(), null, iResultCode.getMessage());
}
private R(IResultCode iResultCode, String msg) {
this(iResultCode.getCode(), null, msg);
}
private R(IResultCode iResultCode, T data) {
this(iResultCode.getCode(), data, iResultCode.getMessage());
}
private R(IResultCode iResultCode, T data, String msg) {
this(iResultCode.getCode(), data, msg);
}
private R(int code, T data, String msg) {
this.code = code;
this.data = data;
this.msg = msg;
this.success = ResultCode.SUCCESS.code == code;
}
public static boolean isSuccess(@Nullable R> result) {
return Optional.ofNullable(result).map((x) -> ObjectUtils.nullSafeEquals(ResultCode.SUCCESS.code, x.code)).orElse(Boolean.FALSE);
}
public static boolean isNotSuccess(@Nullable R> result) {
return !isSuccess(result);
}
public static R data(T data) {
return data(data, ResultCode.SUCCESS.getMessage());
}
public static R data(T data, String msg) {
return data(ResultCode.SUCCESS.code, data, msg);
}
public static R data(int code, T data, String msg) {
return new R(code, data, msg);
}
public static R success() {
return new R(ResultCode.SUCCESS);
}
public static R success(String msg) {
return new R(ResultCode.SUCCESS, msg);
}
private static R success(IResultCode iResultCode) {
return new R(iResultCode);
}
private static R success(IResultCode iResultCode, String msg) {
return new R(iResultCode, msg);
}
public static R fail(String msg) {
return new R(ResultCode.FAILURE, msg);
}
public static R fail(IResultCode iResultCode) {
return new R(iResultCode);
}
public static R fail(IResultCode iResultCode, String msg) {
return new R(iResultCode, msg);
}
public static R fail(int code, String msg) {
return new R(code, null, msg);
}
public static R status(boolean flag) {
return flag ? success("操作成功") : fail("操作失败");
}
public static R status(boolean flag, String msg) {
return flag ? success(msg) : fail("操作失败");
}
public R() {
}
public int getCode() {
return code;
}
public void setCode(int code) {
this.code = code;
}
public T getData() {
return data;
}
public void setData(T data) {
this.data = data;
}
public String getMsg() {
return msg;
}
public void setMsg(String msg) {
this.msg = msg;
}
public boolean isSuccess() {
return success;
}
public void setSuccess(boolean success) {
this.success = success;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy