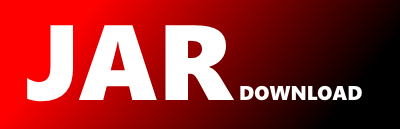
wniemiec.io.java.StandardOutputTerminal Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of terminal Show documentation
Show all versions of terminal Show documentation
Utility for executing commands in a terminal.
The newest version!
/**
* Copyright (c) William Niemiec.
*
* This source code is licensed under the MIT license found in the
* LICENSE file in the root directory of this source tree.
*/
package wniemiec.io.java;
import java.util.ArrayList;
import java.util.List;
import java.util.function.Consumer;
/**
* Standard manager of terminal output.
*/
class StandardOutputTerminal implements OutputTerminal {
//-------------------------------------------------------------------------
// Attributes
//-------------------------------------------------------------------------
private static final Consumer NULL_HANDLER;
private final Consumer outputHandler;
private final Consumer outputErrorHandler;
private final List history;
private final List errorHistory;
//-------------------------------------------------------------------------
// Initialization block
//-------------------------------------------------------------------------
static {
NULL_HANDLER = message -> {};
}
//-------------------------------------------------------------------------
// Constructor
//-------------------------------------------------------------------------
/**
* Standard terminal output manager.
*
* @param outputHandler
* @param outputErrorHandler
*/
public StandardOutputTerminal(
Consumer outputHandler,
Consumer outputErrorHandler
) {
this.outputHandler = parseHandler(outputHandler);
this.outputErrorHandler = parseHandler(outputErrorHandler);
history = new ArrayList<>();
errorHistory = new ArrayList<>();
}
//-------------------------------------------------------------------------
// Methods
//-------------------------------------------------------------------------
private Consumer parseHandler(Consumer handler) {
if (handler == null) {
return NULL_HANDLER;
}
return handler;
}
@Override
public void receive(String message) {
outputHandler.accept(message);
history.add(message);
}
@Override
public void receiveError(String message) {
if (message.isBlank()) {
return;
}
outputErrorHandler.accept(message);
errorHistory.add(message);
}
@Override
public void clear() {
history.clear();
errorHistory.clear();
}
//-------------------------------------------------------------------------
// Getters
//-------------------------------------------------------------------------
@Override
public List getHistory() {
return history;
}
@Override
public List getErrorHistory() {
return errorHistory;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy