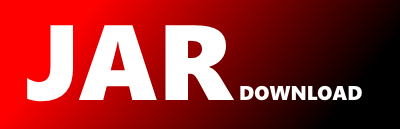
commonMain.com.bselzer.ktx.settings.compose.State.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ktx-settings-compose Show documentation
Show all versions of ktx-settings-compose Show documentation
Extensions for the Kotlin standard library and third-party libraries.
The newest version!
package com.bselzer.ktx.settings.compose
import androidx.compose.runtime.Composable
import androidx.compose.runtime.MutableState
import androidx.compose.runtime.collectAsState
import androidx.compose.runtime.rememberCoroutineScope
import com.bselzer.ktx.settings.setting.Setting
import kotlinx.coroutines.flow.Flow
import kotlinx.coroutines.launch
import kotlin.coroutines.CoroutineContext
import kotlin.coroutines.EmptyCoroutineContext
/**
* Remembers the non-null state of the setting.
*/
@Composable
fun Setting.safeState(coroutineContext: CoroutineContext = EmptyCoroutineContext): MutableState {
val scope = rememberCoroutineScope { coroutineContext }
return object : MutableState {
private val state = observe().collectAsState(defaultValue, coroutineContext)
override var value: T
get() = state.value
set(value) {
scope.launch { set(value) }
}
override fun component1(): T = value
override fun component2(): (T) -> Unit = { value = it }
}
}
/**
* Remembers the nullable state of the setting in a safe way using the default value.
*/
@Composable
fun Setting.defaultState(coroutineContext: CoroutineContext = EmptyCoroutineContext): MutableState =
nullState(observer = { observe() }, initial = defaultValue, coroutineContext = coroutineContext)
/**
* Remembers the nullable state of the setting.
*/
@Composable
fun Setting.nullState(coroutineContext: CoroutineContext = EmptyCoroutineContext): MutableState =
nullState(observer = { observeOrNull() }, initial = null, coroutineContext = coroutineContext)
/**
* Remembers the nullable state of the setting.
*/
@Composable
private fun Setting.nullState(observer: () -> Flow, initial: T?, coroutineContext: CoroutineContext = EmptyCoroutineContext): MutableState {
val scope = rememberCoroutineScope { coroutineContext }
return object : MutableState {
private val state = observer().collectAsState(initial, coroutineContext)
override var value: T?
get() = state.value
set(value) {
scope.launch { if (value == null) remove() else set(value) }
}
override fun component1(): T? = value
override fun component2(): (T?) -> Unit = { value = it }
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy