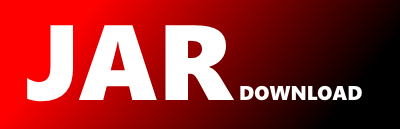
io.github.wycst.wast.common.utils.ObjectUtils Maven / Gradle / Ivy
Show all versions of wast Show documentation
package io.github.wycst.wast.common.utils;
import io.github.wycst.wast.common.beans.DateParser;
import io.github.wycst.wast.common.beans.GregorianDate;
import io.github.wycst.wast.common.exceptions.TypeNotMatchExecption;
import io.github.wycst.wast.common.reflect.*;
import java.lang.reflect.Constructor;
import java.lang.reflect.Method;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.sql.Timestamp;
import java.util.*;
import java.util.concurrent.atomic.AtomicInteger;
import java.util.concurrent.atomic.AtomicLong;
@SuppressWarnings("unchecked")
public final class ObjectUtils extends InvokeUtils {
public static boolean contains(Object target, String key) {
target.getClass();
if (target instanceof Map) {
return ((Map, ?>) target).containsKey(key);
} else {
ClassStructureWrapper classStructureWrapper = ClassStructureWrapper.get(target.getClass());
List getterInfos = classStructureWrapper.getGetterInfos();
for (GetterInfo getterInfo : getterInfos) {
if (getterInfo.getName().equals(key.trim())) {
return true;
}
}
}
return false;
}
/**
* 从上文中读取表达式key
*
* @param context
* @param exprKey
* @param clazz
* @param
* @return
*/
public static E getContext(Object context, String exprKey, Class clazz) {
Object result = get(context, exprKey);
if (result == null) return (E) null;
if (clazz != null && !clazz.isInstance(result)) {
throw new TypeNotMatchExecption(" type is not match, expect " + clazz + ", but get " + result.getClass());
}
return (E) result;
}
public static E get(Object target, String key, Class clazz) {
Object result = get(target, key);
if (result == null) return null;
try {
return (E) result;
} catch (Throwable throwable) {
throw new TypeNotMatchExecption(" type is not match, expect " + clazz + ", but get " + result.getClass());
}
}
/**
* 从对象中查找属性或key对应的value
* 路径中的属性一旦为空返回null
*
* 支持多级访问 eg: user.name
* 支持数组访问 eg: users.[n].name
*
* @param target
* @param key
* @return
*/
public static Object get(Object target, String key) {
if (target == null)
return null;
key = key.trim();
int dotIndex;
if (target instanceof Map) {
Map mapTarget = (Map) target;
Object value = mapTarget.get(key);
if (value != null) {
return value;
}
// 判断是否为多级属性(key中是否包含.),如果是先取一级,递归获取
dotIndex = key.indexOf('.');
if (dotIndex == -1) {
return null;
}
String topKey = key.substring(0, dotIndex);
String nextKey = key.substring(dotIndex + 1);
Object nextTarget = mapTarget.get(topKey);
return get(nextTarget, nextKey);
} else {
dotIndex = key.indexOf('.');
if (dotIndex > -1) {
String topKey = key.substring(0, dotIndex);
String nextKey = key.substring(dotIndex + 1);
Object nextTarget = get(target, topKey);
return get(nextTarget, nextKey);
} else {
if (CollectionUtils.isCollection(target)) {
if ("size".equals(key) || "length".equals(key)) {
return CollectionUtils.getSize(target);
}
if (key.startsWith("[") && key.endsWith("]")) {
return CollectionUtils.getElement(target, Integer.parseInt(key.substring(1, key.length() - 1)));
}
throw new TypeNotMatchExecption("context property '" + key + "' is invalid ");
} else {
return getObjectFieldValue(target, key);
}
}
}
}
/**
* 获取对象的属性值
*
* @param target 对象
* @param field 属性
* @return 返回对象中的属性值
*/
public static Object getAttrValue(Object target, String field) {
if (target == null)
return null;
field.getClass();
if (target instanceof Map) {
return ((Map, ?>) target).get(field);
}
ReflectConsts.ClassCategory classCategory = ReflectConsts.getClassCategory(target.getClass());
switch (classCategory) {
case ObjectCategory: {
return getObjectFieldValue(target, field);
}
case CollectionCategory:
case ArrayCategory: {
if ("size".equals(field) || "length".equals(field)) {
return CollectionUtils.getSize(target);
}
if (field.startsWith("[") && field.endsWith("]")) {
return CollectionUtils.getElement(target, Integer.parseInt(field.substring(1, field.length() - 1)));
}
throw new TypeNotMatchExecption("context property '" + field + "' is invalid ");
}
default: {
return null;
}
}
}
public static Object getObjectFieldValue(Object target, String field) {
ClassStructureWrapper classStructureWrapper = ClassStructureWrapper.get(target.getClass());
GetterInfo getterInfo = classStructureWrapper.getGetterInfo(field);
if (getterInfo != null) {
return getterInfo.invoke(target);
}
return null;
}
/**
* 给目标对象的结构(key)赋值(update)
*
* 支持多级访问 eg: user.name
* 支持数组访问 eg: users.[n].name
*
* @param target
* @param key
* @param value
*/
public static void set(Object target, String key, Object value) {
set(target, key, value, false);
}
/**
* 给目标对象的结构(key)赋值(update)
*
* 支持多级访问 eg: user.name
* 支持数组访问 eg: users.[n].name
*
* @param target
* @param key
* @param value
* @param createIfMapNull 如果路径中属性为空是否创建对象(map属性)
*/
public static void set(Object target, String key, Object value, boolean createIfMapNull) {
if (target == null)
return;
key = key.trim();
/**
* 判断是否为多级属性(key中是否包含.),如果是先取一级,递归获取
*/
int dotIndex = key.indexOf('.');
if (target instanceof Map) {
Map mapTarget = (Map) target;
if (mapTarget.containsKey(key)) {
mapTarget.put(key, value);
} else {
if (dotIndex > -1) {
String topKey = key.substring(0, dotIndex);
String nextKey = key.substring(dotIndex + 1);
Object nextTarget = mapTarget.get(topKey);
if (createIfMapNull && nextTarget == null) {
nextTarget = new LinkedHashMap();
mapTarget.put(topKey, nextTarget);
}
set(nextTarget, nextKey, value, createIfMapNull);
} else {
mapTarget.put(key, value);
}
}
} else {
if (dotIndex > -1) {
String topKey = key.substring(0, dotIndex);
String nextKey = key.substring(dotIndex + 1);
Object nextTarget = get(target, topKey);
set(nextTarget, nextKey, value, createIfMapNull);
} else {
if (CollectionUtils.isCollection(target)) {
if (key.startsWith("[") && key.endsWith("]")) {
CollectionUtils.setElement(target, Integer.parseInt(key.substring(1, key.length() - 1)), value);
}
throw new TypeNotMatchExecption("context property '" + key + "' is invalid ");
} else {
ClassStructureWrapper classStructureWrapper = ClassStructureWrapper.get(target.getClass());
SetterInfo setterInfo = classStructureWrapper.getSetterInfo(key);
if (setterInfo != null) {
invokeSet(setterInfo, target, ObjectUtils.toType(value, setterInfo.getParameterType()));
}
}
}
}
}
public static Object[] get(Object target, List keys) {
Object[] values = new Object[keys.size()];
int index = 0;
for (String key : keys) {
values[index++] = get(target, key);
}
return values;
}
public static Map toMap(Object target) {
if (target == null)
return null;
if (target instanceof Map) {
return (Map) target;
}
Map map = new HashMap();
ClassStructureWrapper classStructureWrapper = ClassStructureWrapper.get(target.getClass());
List getterInfos = classStructureWrapper.getGetterInfos();
for (GetterInfo getterInfo : getterInfos) {
map.put(getterInfo.getName(), invokeGet(getterInfo, target));
}
return map;
}
/**
* 获取对象的非空属性列表
*
* @param target
* @return
*/
public static List getNonEmptyFields(Object target) {
if (target == null)
return null;
List fields = new ArrayList();
if (target instanceof Map) {
Map