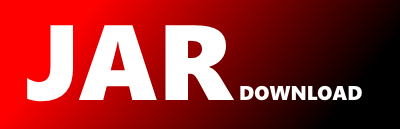
io.github.wycst.wast.jdbc.executer.DefaultSqlExecuter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of wast Show documentation
Show all versions of wast Show documentation
Wast is a high-performance Java toolset library package that includes JSON, YAML, CSV, HttpClient, JDBC and EL engines
package io.github.wycst.wast.jdbc.executer;
import io.github.wycst.wast.jdbc.commands.OperationSqlExecuteCommand;
import io.github.wycst.wast.jdbc.commands.SqlExecuteCall;
import io.github.wycst.wast.jdbc.connection.ConnectionManager;
import io.github.wycst.wast.jdbc.connection.ConnectionWraper;
import io.github.wycst.wast.jdbc.connection.DefaultConnectionManager;
import io.github.wycst.wast.jdbc.dialect.*;
import io.github.wycst.wast.jdbc.exception.SqlExecuteException;
import io.github.wycst.wast.jdbc.interceptor.SqlInterceptor;
import io.github.wycst.wast.jdbc.query.QueryExecutor;
import io.github.wycst.wast.jdbc.query.page.Page;
import io.github.wycst.wast.jdbc.util.SqlUtil;
import io.github.wycst.wast.jdbc.util.StreamCursor;
import io.github.wycst.wast.log.Log;
import io.github.wycst.wast.log.LogFactory;
import javax.sql.DataSource;
import java.io.IOException;
import java.io.InputStream;
import java.io.Serializable;
import java.lang.reflect.Constructor;
import java.sql.*;
import java.util.*;
@SuppressWarnings({"rawtypes", "unchecked"})
public class DefaultSqlExecuter {
private static Log log = LogFactory.getLog(DefaultSqlExecuter.class);
/*唯一id*/
private final String uid = UUID.randomUUID().toString();
/**
* sql执行器属性
*/
private final SqlExecuterProperties executerProperties;
/**
* dataSource
*/
private DataSource dataSource;
/**
* if use spring
*/
private boolean useSpringTransactionManager = false;
/**
* conn manager
*/
private ConnectionManager connectionManager;
// query
private QueryExecutor queryExecutor = new QueryExecutor();
private Dialect dialect;
private PageDialectAgent pageDialectAgent;
private String databaseProductName;
boolean supportBatchInsert;
boolean clickHouse;
boolean mysql;
boolean gbase;
String[] sqlTemplates = new String[SqlFunctionType.values().length];
// api based on sql template
private TemplateSqlExecuter templateExecutor = new TemplateSqlExecuter(this);
// 实体操作
private EntityExecuter entityExecuter = new EntityExecuter(this);
// interceptor
private SqlInterceptor sqlInterceptor;
public DefaultSqlExecuter() {
this(new SqlExecuterProperties());
}
public DefaultSqlExecuter(SqlExecuterProperties executerProperties) {
this.executerProperties = executerProperties == null ? new SqlExecuterProperties() : executerProperties;
}
public void setDataSource(DataSource dataSource) {
dataSource.getClass();
if (dataSource != this.dataSource) {
this.dataSource = dataSource;
this.initDefaultConnectionManager();
this.initialDialect();
}
}
public void setSqlInterceptor(SqlInterceptor sqlInterceptor) {
this.sqlInterceptor = sqlInterceptor;
}
public void setPageDialectAgent(PageDialectAgent pageDialectAgent) {
this.pageDialectAgent = pageDialectAgent;
if (this.dialect != null) {
this.dialect.setPageDialectAgent(pageDialectAgent);
}
}
public DataSource getDataSource() {
return dataSource;
}
private void initDefaultConnectionManager() {
if (this.connectionManager == null) {
// create default if null
this.connectionManager = new DefaultConnectionManager(dataSource);
}
}
public TemplateSqlExecuter getTemplateExecutor() {
return templateExecutor;
}
public EntityExecuter getEntityExecuter() {
return entityExecuter;
}
public void setUseSpringTransactionManager(boolean useSpringTransactionManager) {
this.useSpringTransactionManager = useSpringTransactionManager;
doCreateSpringConnectionManager();
}
public ConnectionManager getConnectionManager() {
return connectionManager;
}
public void setConnectionManager(ConnectionManager connectionManager) {
this.connectionManager = connectionManager;
}
private ConnectionManager currentConnectionManager() {
if (connectionManager == null) {
throw new NullPointerException("ConnectionManager error, Please set the datasource or connectionManager !");
}
return connectionManager;
}
private void doCreateSpringConnectionManager() {
if (useSpringTransactionManager) {
// force to create SpringConnectionManager running at spring env
try {
Class> connectionManagerCls = Class.forName("io.github.wycst.wast.jdbc.spring.connection.SpringConnectionManager");
Constructor> constructor = connectionManagerCls.getConstructor(DataSource.class);
constructor.setAccessible(true);
this.connectionManager = (ConnectionManager) constructor.newInstance(dataSource);
} catch (Exception e) {
e.printStackTrace();
throw new RuntimeException(e);
}
}
}
/**
* @param batchSize
*/
public void setBatchSize(int batchSize) {
executerProperties.setBatchSize(batchSize);
}
public void setQueryTimeout(int queryTimeout) {
executerProperties.setQueryTimeout(queryTimeout);
}
public int getBatchSize() {
return executerProperties.getBatchSize();
}
public void clear() {
currentConnectionManager().clear();
}
public void beginTransaction() {
currentConnectionManager().beginTransaction();
}
/**
* default close connection when commit a transaction
*/
public void commitTransaction() {
commitTransaction(true);
}
/**
* @param closeConnection if close connection
*/
public void commitTransaction(boolean closeConnection) {
currentConnectionManager().commitTransaction(closeConnection);
}
/**
* if end a Transaction ,force close current connection if state of connection is active
*/
public void endTransaction() {
currentConnectionManager().endTransaction();
}
public void rollbackTransaction() {
rollbackTransaction(true);
}
public void rollbackTransaction(boolean closeConnection) {
currentConnectionManager().rollbackTransaction(closeConnection);
}
protected ConnectionWraper getConnectionWraper() {
return currentConnectionManager().getConnectionWraper();
}
Dialect getDialect() {
return dialect;
}
public final String getDatabaseProductName() {
return databaseProductName;
}
public final String getUid() {
return uid;
}
private void initialDialect() {
Connection physicalConn = null;
try {
physicalConn = dataSource.getConnection();
DatabaseMetaData dmd = physicalConn.getMetaData();
String databaseProductName = dmd.getDatabaseProductName();
this.databaseProductName = databaseProductName;
boolean supportBatchInsert = false;
boolean clickHouse = false;
boolean mysql = false;
boolean gbase = false;
if (databaseProductName != null) {
String upperName = databaseProductName.toUpperCase();
if (mysql = (upperName.indexOf("MYSQL") > -1)) {
this.dialect = new MySqlDialect();
} else if (upperName.indexOf("ORACLE") > -1) {
this.dialect = new OracleDialect();
} else if ((clickHouse = upperName.indexOf("CLICKHOUSE") > -1)) {
// ClickHouse
this.dialect = new ClickHouseDialect();
sqlTemplates[SqlFunctionType.UPDATE_BY_ID.ordinal()] = "ALTER TABLE %s UPDATE %s WHERE %s = %s";
sqlTemplates[SqlFunctionType.UPDATE_BY_PARAMS.ordinal()] = "ALTER TABLE %s t UPDATE %s %s";
sqlTemplates[SqlFunctionType.DELETE_BY_ID.ordinal()] = "ALTER TABLE %s DELETE WHERE %s = %s";
sqlTemplates[SqlFunctionType.DELETE_BY_PARAMS.ordinal()] = "ALTER TABLE %s DELETE %s";
} else if (gbase = upperName.indexOf("GBASE") > -1) {
// gbase
this.dialect = new GbaseDialect();
} else {
// default
this.dialect = new DefaultDialect(pageDialectAgent);
}
supportBatchInsert = mysql || clickHouse;
}
this.clickHouse = clickHouse;
this.mysql = mysql;
this.gbase = gbase;
this.supportBatchInsert = supportBatchInsert;
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
if (physicalConn != null) {
try {
physicalConn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
/**
* create connnection of current thread
*
* @return
*/
protected Connection getConnection() {
return getConnectionWraper().getConnection();
}
public boolean isShowSql() {
if (isDevelopment()) {
return true;
}
return executerProperties.getShowSql() == Boolean.TRUE;
}
public void setShowSql(boolean showSql) {
executerProperties.setShowSql(showSql);
}
public boolean isFormatSql() {
return executerProperties.getFormatSql() == Boolean.TRUE;
}
public void setFormatSql(boolean formatSql) {
executerProperties.setFormatSql(formatSql);
}
public boolean isShowParameters() {
if (isDevelopment()) {
return true;
}
return executerProperties.getShowParameters() == Boolean.TRUE;
}
public void setDevelopment(boolean development) {
executerProperties.setDevelopment(development);
}
public boolean isDevelopment() {
return executerProperties.getDevelopment() == Boolean.TRUE;
}
public void setShowSqlParameters(boolean showSqlParameters) {
executerProperties.setShowParameters(showSqlParameters);
}
private E execute(
OperationSqlExecuteCommand command, String sql, Object params, String methodName) {
ConnectionWraper wraper = getConnectionWraper();
E entity = null;
long beginMillis = System.currentTimeMillis();
boolean success = false;
try {
if (sqlInterceptor != null) {
sqlInterceptor.before(sql, params, methodName);
}
if (isShowSql() && sql != null) {
log.info("Sql: {}", sql);
}
if (isShowParameters() && params != null) {
List paramList = getParamList(params);
log.info("Parameters: {}", paramList);
}
// 拦截器设计实现
entity = command.doExecute(wraper);
success = true;
return entity;
} catch (Throwable e) {
throw e instanceof RuntimeException ? (RuntimeException) e : new SqlExecuteException(e.getMessage(), e);
} finally {
if (sqlInterceptor != null) {
sqlInterceptor.after(sql, params, methodName, entity);
}
long endMillis = System.currentTimeMillis();
if (isDevelopment()) {
log.info("- api:[{}], exec: {}ms, success: {}", methodName, endMillis - beginMillis, success);
}
// handler close
if (wraper != null && wraper.autoClose() && command.closeable()) {
connectionManager.closeConnection(wraper);
}
}
}
private List getParamList(Object params) {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy