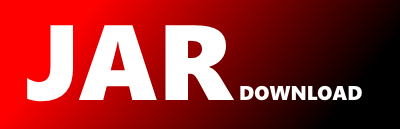
io.github.wycst.wast.jdbc.query.page.Page Maven / Gradle / Ivy
package io.github.wycst.wast.jdbc.query.page;
import java.lang.reflect.ParameterizedType;
import java.lang.reflect.Type;
import java.util.List;
@SuppressWarnings({"unchecked"})
public abstract class Page {
private long page = 1;
private int pageSize = 10;
private List rows;
private long total;
private Long offset;
private Class cls;
public Page() {
}
public Page(Class cls) {
this.cls = cls;
}
public static Page pageInstance(Class cls) {
return new Page(cls) {
};
}
public static Page pageInstance(Class cls, long pageNum, int pageSize) {
Page pageInstance = new Page(cls) {
};
pageInstance.setPageSize(pageSize);
pageInstance.setPage(pageNum);
return pageInstance;
}
public Class actualType() {
if (this.cls != null) {
return cls;
}
ParameterizedType parameterizedType = (ParameterizedType) getClass().getGenericSuperclass();
Type[] types = parameterizedType.getActualTypeArguments();
if (types != null && types.length > 0) {
if (types[0] instanceof Class) {
return (Class) types[0];
}
}
return null;
}
public long getPage() {
return page;
}
public void setPage(long page) {
this.page = page;
}
public int getPageSize() {
return pageSize;
}
public void setPageSize(int pageSize) {
this.pageSize = pageSize;
}
public List getRows() {
return rows;
}
public void setRows(List rows) {
this.rows = rows;
}
public long getTotal() {
return total;
}
public void setTotal(long total) {
this.total = total;
}
public long getCurrentPage() {
return this.page;
}
public long getOffset() {
if (offset == null) {
offset = (page - 1) * pageSize;
}
return offset;
}
public void setOffset(long offset) {
this.offset = offset;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy