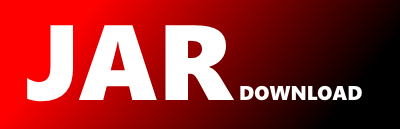
norm.impl.ServiceInterceptor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of norm Show documentation
Show all versions of norm Show documentation
a small java orm library.
package norm.impl;
import net.sf.cglib.proxy.MethodInterceptor;
import net.sf.cglib.proxy.MethodProxy;
import norm.BeanException;
import norm.Norm;
import norm.anno.*;
import norm.anno.cache.Evict;
import norm.anno.cache.EvictAll;
import norm.anno.cache.Put;
import norm.anno.cache.Cache;
import norm.cache.CacheManager;
import ognl.Ognl;
import ognl.OgnlException;
import java.lang.annotation.Annotation;
import java.lang.reflect.Method;
import java.util.HashMap;
import java.util.Map;
public class ServiceInterceptor implements MethodInterceptor {
private Class serviceClass;
private Norm norm;
public ServiceInterceptor(Class serviceClass, Norm norm) {
this.serviceClass = serviceClass;
this.norm = norm;
}
@Override
public Object intercept(Object self, Method thisMethod, Object[] args, MethodProxy proxy) throws Throwable {
if("__getNormObject".equals(thisMethod.getName()) && Norm.class.equals(thisMethod.getReturnType())){
return norm;
}
boolean trans = false;
try {
if(thisMethod.isAnnotationPresent(Transaction.class)){
Transaction transaction = thisMethod.getAnnotation(Transaction.class);
String condition = transaction.condition();
if (condition.isEmpty() || filter(condition, thisMethod, args, null)) {
trans = true;
norm.getTransactional().begin();
}
}
return invoke0(self,thisMethod,proxy,args);
}finally {
if(trans){
norm.getTransactional().commit();
}
}
}
private String createKey(String key,Method method,Object args[],Object result) {
/*if(args.length == 0){
return key;
}*/
if(key.isEmpty()){
return method.getName();
}
Map context = createOgnlContext(method,args);
if(result != null){
context.put("_result_",result);
}
try {
return String.valueOf(Ognl.getValue(key,context));
} catch (OgnlException e) {
throw new BeanException("illegal service "+ serviceClass + ",invalid key expression",e);
}
}
private boolean filter(String condition,Method method,Object args[],Object result) {
if(condition.isEmpty()){
return true;
}
Map context = createOgnlContext(method,args);
if(result != null){
context.put("_result_",result);
}
try {
Object value = Ognl.getValue(condition,context);
if(!(value instanceof Boolean)){
throw new BeanException("illegal service "+ serviceClass + ",condition \"" + condition + "\" should return boolean value.");
}
return (Boolean)value;
} catch (OgnlException e) {
throw new BeanException("illegal service "+ serviceClass + ",invalid key expression",e);
}
}
private Map createOgnlContext(Method method,Object[] args){
Map context = new HashMap();
for(int i=0;i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy