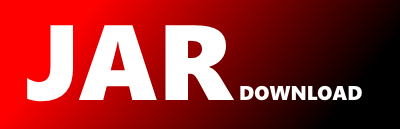
io.github.xezzon.geom.user.UserGrpc Maven / Gradle / Ivy
package io.github.xezzon.geom.user;
import static io.grpc.MethodDescriptor.generateFullMethodName;
/**
*
* 用户相关的服务间接口
*
*/
@javax.annotation.Generated(
value = "by gRPC proto compiler (version 1.64.0)",
comments = "Source: user.proto")
@io.grpc.stub.annotations.GrpcGenerated
public final class UserGrpc {
private UserGrpc() {}
public static final java.lang.String SERVICE_NAME = "io.github.xezzon.geom.user.User";
// Static method descriptors that strictly reflect the proto.
private static volatile io.grpc.MethodDescriptor getAddUserMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "AddUser",
requestType = io.github.xezzon.geom.user.AddUserReq.class,
responseType = io.github.xezzon.geom.user.AddUserResp.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getAddUserMethod() {
io.grpc.MethodDescriptor getAddUserMethod;
if ((getAddUserMethod = UserGrpc.getAddUserMethod) == null) {
synchronized (UserGrpc.class) {
if ((getAddUserMethod = UserGrpc.getAddUserMethod) == null) {
UserGrpc.getAddUserMethod = getAddUserMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "AddUser"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
io.github.xezzon.geom.user.AddUserReq.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
io.github.xezzon.geom.user.AddUserResp.getDefaultInstance()))
.setSchemaDescriptor(new UserMethodDescriptorSupplier("AddUser"))
.build();
}
}
}
return getAddUserMethod;
}
/**
* Creates a new async stub that supports all call types for the service
*/
public static UserStub newStub(io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public UserStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new UserStub(channel, callOptions);
}
};
return UserStub.newStub(factory, channel);
}
/**
* Creates a new blocking-style stub that supports unary and streaming output calls on the service
*/
public static UserBlockingStub newBlockingStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public UserBlockingStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new UserBlockingStub(channel, callOptions);
}
};
return UserBlockingStub.newStub(factory, channel);
}
/**
* Creates a new ListenableFuture-style stub that supports unary calls on the service
*/
public static UserFutureStub newFutureStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public UserFutureStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new UserFutureStub(channel, callOptions);
}
};
return UserFutureStub.newStub(factory, channel);
}
/**
*
* 用户相关的服务间接口
*
*/
public interface AsyncService {
/**
*
* 新增用户
*
*/
default void addUser(io.github.xezzon.geom.user.AddUserReq request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getAddUserMethod(), responseObserver);
}
}
/**
* Base class for the server implementation of the service User.
*
* 用户相关的服务间接口
*
*/
public static abstract class UserImplBase
implements io.grpc.BindableService, AsyncService {
@java.lang.Override public final io.grpc.ServerServiceDefinition bindService() {
return UserGrpc.bindService(this);
}
}
/**
* A stub to allow clients to do asynchronous rpc calls to service User.
*
* 用户相关的服务间接口
*
*/
public static final class UserStub
extends io.grpc.stub.AbstractAsyncStub {
private UserStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected UserStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new UserStub(channel, callOptions);
}
/**
*
* 新增用户
*
*/
public void addUser(io.github.xezzon.geom.user.AddUserReq request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getAddUserMethod(), getCallOptions()), request, responseObserver);
}
}
/**
* A stub to allow clients to do synchronous rpc calls to service User.
*
* 用户相关的服务间接口
*
*/
public static final class UserBlockingStub
extends io.grpc.stub.AbstractBlockingStub {
private UserBlockingStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected UserBlockingStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new UserBlockingStub(channel, callOptions);
}
/**
*
* 新增用户
*
*/
public io.github.xezzon.geom.user.AddUserResp addUser(io.github.xezzon.geom.user.AddUserReq request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getAddUserMethod(), getCallOptions(), request);
}
}
/**
* A stub to allow clients to do ListenableFuture-style rpc calls to service User.
*
* 用户相关的服务间接口
*
*/
public static final class UserFutureStub
extends io.grpc.stub.AbstractFutureStub {
private UserFutureStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected UserFutureStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new UserFutureStub(channel, callOptions);
}
/**
*
* 新增用户
*
*/
public com.google.common.util.concurrent.ListenableFuture addUser(
io.github.xezzon.geom.user.AddUserReq request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getAddUserMethod(), getCallOptions()), request);
}
}
private static final int METHODID_ADD_USER = 0;
private static final class MethodHandlers implements
io.grpc.stub.ServerCalls.UnaryMethod,
io.grpc.stub.ServerCalls.ServerStreamingMethod,
io.grpc.stub.ServerCalls.ClientStreamingMethod,
io.grpc.stub.ServerCalls.BidiStreamingMethod {
private final AsyncService serviceImpl;
private final int methodId;
MethodHandlers(AsyncService serviceImpl, int methodId) {
this.serviceImpl = serviceImpl;
this.methodId = methodId;
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public void invoke(Req request, io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
case METHODID_ADD_USER:
serviceImpl.addUser((io.github.xezzon.geom.user.AddUserReq) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
default:
throw new AssertionError();
}
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public io.grpc.stub.StreamObserver invoke(
io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
default:
throw new AssertionError();
}
}
}
public static final io.grpc.ServerServiceDefinition bindService(AsyncService service) {
return io.grpc.ServerServiceDefinition.builder(getServiceDescriptor())
.addMethod(
getAddUserMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
io.github.xezzon.geom.user.AddUserReq,
io.github.xezzon.geom.user.AddUserResp>(
service, METHODID_ADD_USER)))
.build();
}
private static abstract class UserBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoFileDescriptorSupplier, io.grpc.protobuf.ProtoServiceDescriptorSupplier {
UserBaseDescriptorSupplier() {}
@java.lang.Override
public com.google.protobuf.Descriptors.FileDescriptor getFileDescriptor() {
return io.github.xezzon.geom.user.UserProto.getDescriptor();
}
@java.lang.Override
public com.google.protobuf.Descriptors.ServiceDescriptor getServiceDescriptor() {
return getFileDescriptor().findServiceByName("User");
}
}
private static final class UserFileDescriptorSupplier
extends UserBaseDescriptorSupplier {
UserFileDescriptorSupplier() {}
}
private static final class UserMethodDescriptorSupplier
extends UserBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoMethodDescriptorSupplier {
private final java.lang.String methodName;
UserMethodDescriptorSupplier(java.lang.String methodName) {
this.methodName = methodName;
}
@java.lang.Override
public com.google.protobuf.Descriptors.MethodDescriptor getMethodDescriptor() {
return getServiceDescriptor().findMethodByName(methodName);
}
}
private static volatile io.grpc.ServiceDescriptor serviceDescriptor;
public static io.grpc.ServiceDescriptor getServiceDescriptor() {
io.grpc.ServiceDescriptor result = serviceDescriptor;
if (result == null) {
synchronized (UserGrpc.class) {
result = serviceDescriptor;
if (result == null) {
serviceDescriptor = result = io.grpc.ServiceDescriptor.newBuilder(SERVICE_NAME)
.setSchemaDescriptor(new UserFileDescriptorSupplier())
.addMethod(getAddUserMethod())
.build();
}
}
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy