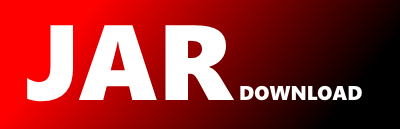
com.zlyx.easy.database.interceptors.AbstractResultSetInterceptor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of easy-database Show documentation
Show all versions of easy-database Show documentation
Base tool for database access.
package com.zlyx.easy.database.interceptors;
import java.lang.reflect.Field;
import java.lang.reflect.Modifier;
import java.math.BigInteger;
import java.sql.ResultSet;
import java.sql.ResultSetMetaData;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import com.zlyx.easy.core.map.Maps;
import com.zlyx.easy.core.reflect.FieldUtils;
import com.zlyx.easy.core.tool.CamelTool;
import com.zlyx.easy.core.utils.ObjectUtils;
import com.zlyx.easy.database.local.ReturnType;
import com.zlyx.easy.database.utils.TableUtils;
/**
* @Auth 赵光
* @Describle
* @2019年1月14日 上午9:57:50
*/
public abstract class AbstractResultSetInterceptor {
@SuppressWarnings({ "rawtypes", "unchecked" })
protected List getResults(Class> resultType, ResultSet resultSet) throws SQLException {
ResultSetMetaData rsmd = resultSet.getMetaData();
List resList = new ArrayList();
Map fieldAndValues = null;
Object value = null;
while (resultSet.next()) {
fieldAndValues = Maps.newMap();
for (int i = 0; i < rsmd.getColumnCount(); i++) {
value = resultSet.getObject(i + 1);
if (value != null) {
fieldAndValues.put(rsmd.getColumnLabel(i + 1).toLowerCase(), value);
}
}
resList.add(packageModel(fieldAndValues, resultType));
}
resultSet.close();
ReturnType.clear();
return resList;
}
/**
* 数据转实体
*
* @param map
* @param c
* @return
*/
public Object packageModel(Map map, Class> resultType) {
boolean notNull = false;
try {
List fields = FieldUtils.getAllFields(resultType);
Object obj = resultType.getConstructor().newInstance();
String tableAlias = TableUtils.getTableName(resultType);
Object value = null;
for (Field field : fields) {
if (!Modifier.isStatic(field.getModifiers()) && !Modifier.isFinal(field.getModifiers())) {
if (!field.getType().toString().contains("java")) {
value = this.packageModel(map, field.getType());
} else if (map.containsKey(tableAlias + "_" + field.getName())) {
value = map.get(tableAlias + "_" + field.getName());
} else if (map.containsKey(TableUtils.getColumnName(field))) {
value = map.get(TableUtils.getColumnName(field));
} else if (map.containsKey(CamelTool.camel2Underline(field.getName()))) {
value = map.get(CamelTool.camel2Underline(field.getName()));
} else {
value = null;
}
if (ObjectUtils.isNotEmpty(value)) {
if (BigInteger.class == value.getClass() && Long.class == field.getType()) {
value = Long.parseLong(String.valueOf(value));
}
if (String.class == field.getType()) {
value = String.valueOf(value);
}
if (Integer.class == field.getType()) {
value = Integer.parseInt(String.valueOf(value));
}
field.setAccessible(true);
field.set(obj, value);
notNull = true;
}
}
}
if (notNull) {
return obj;
}
} catch (Exception e) {
return map;
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy