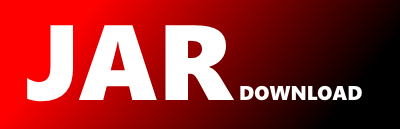
com.zlyx.easy.netty.client.NettyUtils Maven / Gradle / Ivy
package com.zlyx.easy.netty.client;
import java.util.Arrays;
import com.zlyx.easy.core.loggers.Logger;
import com.zlyx.easy.core.utils.ByteUtils;
import io.netty.bootstrap.Bootstrap;
import io.netty.channel.Channel;
import io.netty.channel.ChannelFuture;
import io.netty.channel.ChannelHandler.Sharable;
import io.netty.channel.ChannelHandlerContext;
import io.netty.channel.ChannelInboundHandlerAdapter;
import io.netty.channel.ChannelInitializer;
import io.netty.channel.ChannelOption;
import io.netty.channel.EventLoopGroup;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.SocketChannel;
import io.netty.channel.socket.nio.NioSocketChannel;
import io.netty.handler.codec.bytes.ByteArrayDecoder;
import io.netty.handler.codec.bytes.ByteArrayEncoder;
import io.netty.util.ReferenceCountUtil;
/**
*
* @Auth 赵光
* @Desc 描述 Netty非阻塞式->短连接->客户端
* @Date 2019年4月8日
*/
@Sharable
public class NettyUtils extends ChannelInboundHandlerAdapter {
private boolean isFinish;
private byte[] res;
private static NettyUtils client;
private String host;
private int port;
private Bootstrap b;
private ChannelFuture cf;
private EventLoopGroup group;
private NettyUtils(String host, int port) {
this.group = new NioEventLoopGroup();
this.b = new Bootstrap();
this.b.group(group).channel(NioSocketChannel.class).option(ChannelOption.TCP_NODELAY, true)
.handler(new ChannelInitializer() {
@Override
protected void initChannel(SocketChannel socketChannel) throws Exception {
socketChannel.pipeline().addLast("decoder", new ByteArrayDecoder());
socketChannel.pipeline().addLast("channelHandler", NettyUtils.this);
socketChannel.pipeline().addLast("encoder", new ByteArrayEncoder());
}
});
this.host = host;
this.port = port;
}
/**
* 发送byte数组
*
* @param msg
* @throws InterruptedException
*/
public void sendByteMsg(byte[] msg) throws InterruptedException {
if (msg == null) {
Logger.warn("消息内容为空!");
return;
}
Channel channel = null;
try {
isFinish = false;
channel = getChannel();
channel.writeAndFlush(msg);
channel.closeFuture().sync();
} catch (InterruptedException e) {
e.printStackTrace();
} finally {
if (channel != null) {
channel.close();
}
}
}
/**
* 发送byte数组
*
* @param msg
* @param millis
* @return
* @throws InterruptedException
* @throws Exception
*/
public byte[] sendByteMsg(byte[] msg, int millis) throws InterruptedException {
if (msg != null) {
Logger.info("Netty发送消息 :{}", Arrays.toString(msg));
sendByteMsg(msg);
if (millis != 0) {
do {
Thread.sleep(millis);
} while (!isFinish);
Logger.info("Netty接受结果 :{}", res);
return res;
}
}
return null;
}
/**
* 发送16进制字符串
*
* @param hex 待发送十六进制字符串
* @param millis 等待结果期间阻塞时线程休眠间隔(置为0时不会等待响应结果就返回)
* @return 返回十六进制字符串
* @throws InterruptedException
*/
public String sendHexMsg(String hex, int millis) throws InterruptedException {
if (hex != null && hex != "") {
byte[] msg = ByteUtils.hexStringToByte(hex);
byte[] res = sendByteMsg(msg, millis);
if (res != null) {
return ByteUtils.bytesToHexString(res);
}
}
return null;
}
/**
* 发送16进制字符串
*
* @param hex 待发送十六进制字符串
* @return
* @throws InterruptedException
*/
public void sendHexMsg(String hex) throws InterruptedException {
sendHexMsg(hex, 0);
}
/**
* 发送非16进制字符串
*
* @param hex 待发送非十六进制字符串
* @param millis 等待结果期间阻塞时线程休眠间隔(置为0时不会等待响应结果就返回)
* @return 返回非十六进制字符串
* @throws InterruptedException
*/
public String sendMsg(String msg, int millis) throws InterruptedException {
if (msg != null && msg != "") {
byte[] res = sendByteMsg(msg.getBytes(), millis);
if (res != null) {
return new String(res);
}
}
return null;
}
/**
* 发送非16进制字符串
*
* @param msg
* @return
* @throws InterruptedException
*/
public void sendMsg(String msg) throws InterruptedException {
sendMsg(msg, 0);
}
private Channel getChannel() {
if (this.cf == null) {
try {
this.cf = b.connect(host, port).sync();
} catch (InterruptedException e) {
e.printStackTrace();
}
} else if (!this.cf.channel().isActive()) {
try {
this.cf = b.connect(host, port).sync();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
return this.cf.channel();
}
public static NettyUtils getInstance(String host, int port) {
if (client == null) {
client = new NettyUtils(host, port);
}
return client;
}
@Override
public void channelRead(ChannelHandlerContext ctx, Object msg) throws Exception {
try {
this.res = (byte[]) msg;
} finally {
ReferenceCountUtil.release(msg);
}
}
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) throws Exception {
ctx.close();
}
@Override
public void channelReadComplete(ChannelHandlerContext ctx) throws Exception {
ctx.flush();
ctx.channel().close();
isFinish = true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy