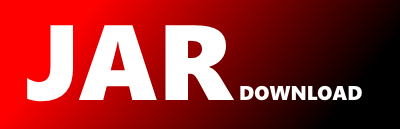
io.github.yamlpath.WorkUnit Maven / Gradle / Ivy
package io.github.yamlpath;
import static io.github.yamlpath.utils.PathUtils.DOT;
import static io.github.yamlpath.utils.PathUtils.ESCAPE;
import static io.github.yamlpath.utils.PathUtils.NO_REPLACEMENT;
import static io.github.yamlpath.utils.PathUtils.PARENTHESIS_CLOSE;
import static io.github.yamlpath.utils.PathUtils.PARENTHESIS_OPEN;
import static io.github.yamlpath.utils.PathUtils.normalize;
import java.util.Map;
import io.github.yamlpath.setters.LastNodeSetter;
import io.github.yamlpath.setters.Setter;
import io.github.yamlpath.utils.StringUtils;
public class WorkUnit {
private String expression;
private Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy