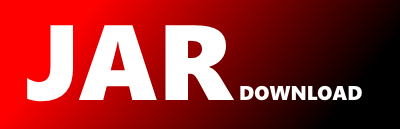
edi.rule.work.processor.JSSAXSheetContent Maven / Gradle / Ivy
The newest version!
package edi.rule.work.processor;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import edi.rule.util.ZSVessel;
import org.apache.poi.xssf.eventusermodel.XSSFSheetXMLHandler.SheetContentsHandler;
import org.apache.poi.xssf.usermodel.XSSFComment;
import edi.rule.config.JSRuleContext;
import edi.rule.core.JSRuleDBCompatible;
import edi.rule.model.JSRuleAction;
import edi.rule.model.JSRuleImportSheet;
import edi.rule.work.constant.JSRuleJsonConfig;
import edi.rule.work.custom.JSRuleException;
import lombok.Data;
/**
* @author 摩拉克斯
* @date 2022年7月8日 上午10:30:40
*/
@Data
public final class JSSAXSheetContent> implements SheetContentsHandler{
public final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy