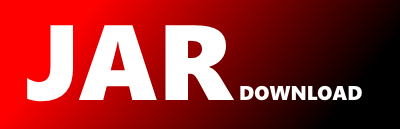
com.zhangyue.we.anoprocesser.xml.StyleReader Maven / Gradle / Ivy
The newest version!
package com.zhangyue.we.anoprocesser.xml;
import com.zhangyue.we.anoprocesser.FileFilter;
import com.zhangyue.we.anoprocesser.Log;
import org.xml.sax.Attributes;
import org.xml.sax.SAXException;
import org.xml.sax.helpers.DefaultHandler;
import java.io.File;
import java.util.ArrayList;
import java.util.HashMap;
import javax.xml.parsers.SAXParser;
import javax.xml.parsers.SAXParserFactory;
/**
* @author chengwei 2018/8/15
*/
public class StyleReader {
private HashMap mStyles;
private File mRootFile;
private SAXParser mParser;
public StyleReader(File file, HashMap styles) {
this.mStyles = styles;
this.mRootFile = findRoot(file);
try {
this.mParser = SAXParserFactory.newInstance().newSAXParser();
} catch (Exception e) {
e.printStackTrace();
}
}
private File findRoot(File file) {
File rootFile = file;
while (!rootFile.getName().equals(Constants.PROJECT_NAME_KMO)) {
rootFile = rootFile.getParentFile();
}
return rootFile;
}
public void parse() {
long start = System.currentTimeMillis();
HashMap> styles = scanStyles(mRootFile);
Log.w("scanStyles take: " + (System.currentTimeMillis() - start));
Log.w("parse " + mRootFile.getAbsolutePath());
for (ArrayList list : styles.values()) {
for (File file : list) {
try {
Log.w("style " + file.getAbsolutePath());
mParser.parse(file, new StyleHandler());
} catch (Exception e) {
e.printStackTrace();
}
}
}
}
private HashMap> scanStyles(File root) {
return new FileFilter(root)
.include("values")
.fileStart("style")
.exclude("layout")
.exclude("build")
.exclude("gradleBuild")
.exclude("java")
.exclude("libs")
.exclude("mipmap")
.exclude("drawable")
.exclude("anim")
.exclude("color")
.exclude("menu")
.exclude("raw")
.exclude("xml")
.filter();
}
private class StyleHandler extends DefaultHandler {
private Style mStyle;
private String mName;
private StringBuilder stringBuilder;
@Override
public void startDocument() throws SAXException {
super.startDocument();
}
@Override
public void startElement(String uri, String localName, String qName, Attributes attributes) throws SAXException {
super.startElement(uri, localName, qName, attributes);
mName = attributes.getValue("name");
if (qName.equals("style")) {
mStyle = new Style();
mStyle.name = mName;
String parent = attributes.getValue("parent");
if (parent != null) {
parent = parent.substring(parent.indexOf("/") + 1);
}
mStyle.parent = parent;
if (mStyle.parent == null && mName.contains(".")) {
mStyle.parent = mName.substring(0, mName.lastIndexOf("."));
}
} else if (qName.equals("item")) {
if (mStyle != null) {
mStyle.attribute.put(mName, localName);
stringBuilder = new StringBuilder();
}
}
}
@Override
public void characters(char[] ch, int start, int length) throws SAXException {
super.characters(ch, start, length);
if (stringBuilder != null) {
stringBuilder.append(ch, start, length);
}
}
@Override
public void endElement(String uri, String localName, String qName) throws SAXException {
super.endElement(uri, localName, qName);
if (qName.equals("style")) {
mStyles.put(mStyle.name, mStyle);
mStyle = null;
} else if (qName.equals("item")) {
if (mStyle != null) {
mStyle.attribute.put(mName, stringBuilder.toString());
}
stringBuilder = null;
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy