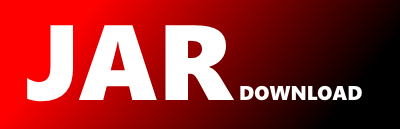
io.milvus.response.DescCollResponseWrapper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of milvus-sdk-java Show documentation
Show all versions of milvus-sdk-java Show documentation
Java SDK for Milvus, a distributed high-performance vector search engine.
update grpc to 1.42.1
update protobuf to 3.19.1
restore the calcDistance interface that is removed in 2.1.0-beta4
package io.milvus.response;
import io.milvus.grpc.CollectionSchema;
import io.milvus.grpc.DescribeCollectionResponse;
import io.milvus.grpc.FieldSchema;
import io.milvus.param.ParamUtils;
import io.milvus.param.collection.FieldType;
import lombok.NonNull;
import java.util.ArrayList;
import java.util.List;
/**
* Util class to wrap response of describeCollection
interface.
*/
public class DescCollResponseWrapper {
private final DescribeCollectionResponse response;
public DescCollResponseWrapper(@NonNull DescribeCollectionResponse response) {
this.response = response;
}
/**
* Get name of the collection.
*
* @return String
name of the collection
*/
public String getCollectionName() {
CollectionSchema schema = response.getSchema();
return schema.getName();
}
/**
* Get description of the collection.
*
* @return String
description of the collection
*/
public String getCollectionDescription() {
CollectionSchema schema = response.getSchema();
return schema.getDescription();
}
/**
* Get internal id of the collection.
*
* @return long
internal id of the collection
*/
public long getCollectionID() {
return response.getCollectionID();
}
/**
* Get shard number of the collection.
*
* @return int
shard number of the collection
*/
public int getShardNumber() {
return response.getShardsNum();
}
/**
* Get utc timestamp when collection created.
*
* @return long
utc timestamp when collection created
*/
public long getCreatedUtcTimestamp() {
return response.getCreatedUtcTimestamp();
}
/**
* Get aliases of the collection.
*
* @return List of String, aliases of the collection
*/
public List getAliases() {
List aliases = new ArrayList<>();
for (int i = 0; i < response.getAliasesCount(); ++i) {
aliases.add(response.getAliases(i));
}
return aliases;
}
/**
* Get schema of the collection's fields.
*
* @return List of FieldType, schema of the collection's fields
*/
public List getFields() {
List results = new ArrayList<>();
CollectionSchema schema = response.getSchema();
List fields = schema.getFieldsList();
fields.forEach((field) -> results.add(ParamUtils.ConvertField(field)));
return results;
}
/**
* Get schema of a field by name.
* Return null if the field doesn't exist
*
* @param fieldName field name to get field description
* @return {@link FieldType} schema of the field
*/
public FieldType getFieldByName(@NonNull String fieldName) {
CollectionSchema schema = response.getSchema();
for (int i = 0; i < schema.getFieldsCount(); ++i) {
FieldSchema field = schema.getFields(i);
if (fieldName.compareTo(field.getName()) == 0) {
return ParamUtils.ConvertField(field);
}
}
return null;
}
/**
* Construct a String
by {@link DescCollResponseWrapper} instance.
*
* @return String
*/
@Override
public String toString() {
return "Collection Description{" +
"name:'" + getCollectionName() + '\'' +
", description:'" + getCollectionDescription() + '\'' +
", id:" + getCollectionID() +
", shardNumber:" + getShardNumber() +
", createdUtcTimestamp:" + getCreatedUtcTimestamp() +
", aliases:" + getAliases().toString() +
", fields:" + getFields().toString() +
'}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy