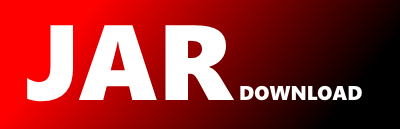
acm.graphics.GPoint Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of javakarel Show documentation
Show all versions of javakarel Show documentation
This the original Stanford Karel for Java, packaged for Maven. ACM Library is included. See also https://cs.stanford.edu/people/eroberts/karel-the-robot-learns-java.pdf
The newest version!
/*
* @(#)GPoint.java 1.99.1 08/12/08
*/
// ************************************************************************
// * Copyright (c) 2008 by the Association for Computing Machinery *
// * *
// * The Java Task Force seeks to impose few restrictions on the use of *
// * these packages so that users have as much freedom as possible to *
// * use this software in constructive ways and can make the benefits of *
// * that work available to others. In view of the legal complexities *
// * of software development, however, it is essential for the ACM to *
// * maintain its copyright to guard against attempts by others to *
// * claim ownership rights. The full text of the JTF Software License *
// * is available at the following URL: *
// * *
// * http://www.acm.org/jtf/jtf-software-license.pdf *
// * *
// ************************************************************************
// REVISION HISTORY
//
// -- V2.0 --
// Performance improvement 23-Jan-07 (ESR)
// 1. Changed hashCode implementation for better performance.
//
// Feature enhancement 26-May-08 (ESR)
// 1. Added support for serialization.
package acm.graphics;
import java.awt.*;
import java.io.*;
/* Class: GPoint */
/**
* This class is a double-precision version of the Point
class
* in java.awt
.
*/
public class GPoint implements Serializable {
/* Constructor: GPoint() */
/**
* Constructs a new GPoint
at the origin (0, 0).
*
* Example: pt = new GPoint();
*/
public GPoint() {
this(0, 0);
}
/* Constructor: GPoint(x, y) */
/**
* Constructs a new GPoint
with the specified coordinates.
*
* Example: pt = new GPoint(x, y);
* @param x The x-coordinate of the point
* @param y The y-coordinate of the point
*/
public GPoint(double x, double y) {
xc = x;
yc = y;
}
/* Constructor: GPoint(p) */
/**
* Constructs a new GPoint
from an existing one.
*
* Example: pt = new GPoint(p);
* @param p The original point
*/
public GPoint(GPoint p) {
this(p.xc, p.yc);
}
/* Constructor: GPoint(p) */
/**
* Constructs a new GPoint
from an existing AWT Point
.
*
* Example: pt = new GPoint(p);
* @param p An AWT Point
*/
public GPoint(Point p) {
this(p.x, p.y);
}
/* Method: getX() */
/**
* Returns the x coordinate of this GPoint
.
*
* Example: x = pt.getX();
* @return The x coordinate of this GPoint
*/
public double getX() {
return xc;
}
/* Method: getY() */
/**
* Returns the y coordinate of this GPoint
.
*
* Example: y = pt.getY();
* @return The y coordinate of this GPoint
*/
public double getY() {
return yc;
}
/* Method: setLocation(x, y) */
/**
* Sets the location of the GPoint
to the specified x
* and y
values.
*
* Example: pt.setLocation(x, y);
* @param x The new x-coordinate for the point
* @param y The new y-coordinate for the point
*/
public void setLocation(double x, double y) {
xc = x;
yc = y;
}
/* Method: setLocation(p) */
/**
* Sets the location of the GPoint
to that of an existing one.
*
* Example: pt.setLocation(p);
* @param p An existing GPoint
specifying the new location
*/
public void setLocation(GPoint p) {
setLocation(p.xc, p.yc);
}
/* Method: getLocation() */
/**
* Returns a new GPoint
whose coordinates are the same as this one.
*
* Example: p = pt.getLocation();
* @return A new point with the same coordinates
*/
public GPoint getLocation() {
return new GPoint(xc, yc);
}
/* Method: translate(dx, dy) */
/**
* Adjusts the coordinates of a point by the specified dx
and
* dy
offsets.
*
* Example: pt.translate(dx, dy);
* @param dx The change in the x direction (positive is rightward)
* @param dy The change in the y direction (positive is downward)
*/
public void translate(double dx, double dy) {
xc += dx;
yc += dy;
}
/* Method: toPoint() */
/**
* Converts this GPoint
to the nearest integer-based Point
.
*
* Example: size = dim.toPoint();
* @return The closest integer-based Point
*/
public Point toPoint() {
return new Point((int) Math.round(xc), (int) Math.round(yc));
}
/* Method: hashCode() */
/**
* Returns an integer hash code for the point. The hash code for a
* GPoint
is constructed from the hash codes from the
* float
values of the coordinates, which are the ones used in the
* equals
method.
*
* Example: hash = pt.hashCode();
* @return The hash code for this pt
*
*/
public int hashCode() {
return new Float((float) xc).hashCode() ^ (37 * new Float((float) yc).hashCode());
}
/* Method: equals(obj) */
/**
* Tests whether two GPoint
objects are equal.
* Because floating-point values are inexact, this method checks for
* equality by comparing the float
values (rather than the
* double
values) of the coordinates.
*
* Example: if (pt.equals(obj)) . . .
* @param obj Any object
* @return true
if the obj
is a GPoint
* equal to this one, and false
otherwise
*
*/
public boolean equals(Object obj) {
if (!(obj instanceof GPoint)) return false;
GPoint pt = (GPoint) obj;
return ((float) xc == (float) pt.xc) && ((float) yc == (float) pt.yc);
}
/* Method: toString() */
/**
* Converts this GPoint
to its string representation.
*
* Example: str = rect.toString();
* @return A string representation of this point
*
*/
public String toString() {
return "(" + (float) xc + ", " + (float) yc + ")";
}
/* Private instance variables */
private double xc;
private double yc;
/* Serial version UID */
/**
* The serialization code for this class. This value should be incremented
* whenever you change the structure of this class in an incompatible way,
* typically by adding a new instance variable.
*/
static final long serialVersionUID = 1L;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy