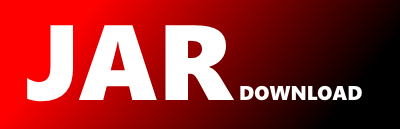
acm.program.package.html Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of javakarel Show documentation
Show all versions of javakarel Show documentation
This the original Stanford Karel for Java, packaged for Maven. ACM Library is included. See also https://cs.stanford.edu/people/eroberts/karel-the-robot-learns-java.pdf
The newest version!
The acm.program Package
This package provides a set of classes that simplify the creation of programs.
The package includes five abstract classes, each of which uses a particular
paradigm for input and output, as follows:
Program
This class is the root of the program hierachy and defines a program in which
input and output are performed using the system console, which is redirected to
the standard Java I/O streams System.in
and System.out
.
ConsoleProgram
This class is similar to Program
but
fills the program frame with an interactive console (as defined in the
IOConsole
class in the
acm.io
package).
DialogProgram
This class extends the basic functionality of the Program
class so that input operations are performed using the
IODialog
class in the
acm.io
package.
CommandLineProgram
This class is similar to
ConsoleProgram
but uses System.in
and System.out
instead of
an IOConsole
. This class is typically used only for Java
programs that are invoked from a command line and make no use of Javas
graphical capabilities.
The principal advantages of using the Program
class are:
- The conventional pattern of use associated with the
acm.program
package moves
students away from the imperative style of public static void main
into a more pedagogically defensible framework in which students are always working in the
context of an object.
- The
Program
class allows Java applications to double as applets,
thereby making it possible for intructors to use either paradigm in a consistent way.
Even for those instructors that choose to focus on the application paradigm, using
the Program
class makes it much easier for students to make their code
available on the web. Moreover, because the Program
class is defined
to be a subclass of Applet
, applications that run in that domain can
take advantage of such applet-based features as loading audio clips and images from
the code base.
- The
Program
class includes several features that make instruction
easier, such as the definition of standard menu bars that support operations like
printing and running programs with a test script.
- The classes in the
acm.program
package offer a compelling example
of an inheritance hierarchy that introductory students can understand and appreciate
right from the beginning of their first course.
In most environments, the only thing students need to do to create a new
program is to define a Program
subclass that implements a run
method. For example, the following class definition creates a traditional,
stream-oriented program that reads in two integers and computes their sum:
public class Add2 extends ConsoleProgram {
public void run() {
println("This program adds two numbers.");
int n1 = readInt("Enter n1: ");
int n2 = readInt("Enter n2: ");
int total = n1 + n2;
println("The total is " + total + ".");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy