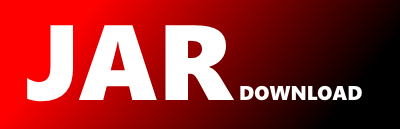
io.zero88.jooqx.BindBatchValues Maven / Gradle / Ivy
The newest version!
package io.zero88.jooqx;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.stream.Collectors;
import org.jooq.Field;
import org.jooq.InsertSetStep;
import org.jooq.MergeMatchedSetStep;
import org.jooq.Name;
import org.jooq.Record;
import org.jooq.UpdateSetStep;
import lombok.NonNull;
/**
* Represents a holder keep dummy value and list of binding records
*
* @apiNote With {@code dummy value records}, you can predefine a field value, then if a bind record is missing this
* field value then system will auto detect and fallback to the predefined value. See: {@link #registerValue(Field,
* Object)}
* @see JDBC batch operations
* @see InsertSetStep#set(Map)
* @see UpdateSetStep#set(Map)
* @see MergeMatchedSetStep#set(Map)
* @since 1.0.0
*/
public final class BindBatchValues {
private final Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy