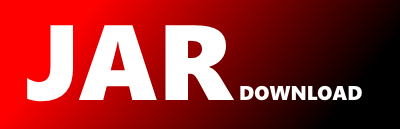
io.zero88.jooqx.DSLAdapter Maven / Gradle / Ivy
The newest version!
package io.zero88.jooqx;
import java.util.Collection;
import org.jooq.Field;
import org.jooq.Record;
import org.jooq.Record1;
import org.jooq.Table;
import org.jooq.TableLike;
import org.jooq.TableRecord;
import io.zero88.jooqx.adapter.SQLResultAdapter;
import io.zero88.jooqx.adapter.SelectCount;
import io.zero88.jooqx.adapter.SelectExists;
import io.zero88.jooqx.adapter.SelectList;
import io.zero88.jooqx.adapter.SelectOne;
import lombok.NonNull;
/**
* DSL adapter
*
* @since 1.0.0
*/
public interface DSLAdapter {
/**
* Fetch count
*
* @param table a query table context
* @return select count
*/
static SelectCount fetchCount(@NonNull TableLike> table) {
return new SelectCount(table);
}
/**
* Fetch exists
*
* @param table a query table context
* @return select exists
*/
static SelectExists fetchExists(@NonNull TableLike> table) {
return new SelectExists(table);
}
/**
* Fetch one JsonRecord
*
* @param table a query table context
* @param Type of jOOQ Table in Query context
* @return select one adapter
* @see TableLike
* @see JsonRecord
*/
static > SelectOne, JsonRecord>> fetchJsonRecord(
@NonNull T table) {
return new SelectOne<>(table, SQLResultAdapter.byJson());
}
/**
* Fetch one
*
* @param table a query table context
* @param record record
* @param Type of jOOQ Table in Query context
* @param Type of output jOOQ record
* @return select one adapter
* @see TableLike
*/
static , R extends TableRecord> SelectOne fetchOne(
@NonNull T table, @NonNull R record) {
return new SelectOne<>(table, SQLResultAdapter.byRecord(record));
}
/**
* Fetch one
*
* @param table a query table context
* @param fields given fields
* @param Type of jOOQ Table in Query context
* @return select one adapter
* @see TableLike
*/
static > SelectOne fetchOne(@NonNull T table,
@NonNull Collection> fields) {
return new SelectOne<>(table, SQLResultAdapter.byFields(fields));
}
/**
* Fetch one
*
* @param table a query table context
* @param outputClass given output class
* @param Type of jOOQ Table in Query context
* @param Type ot output class
* @return select one adapter
* @see TableLike
*/
static , R> SelectOne, R> fetchOne(@NonNull T table,
@NonNull Class outputClass) {
return new SelectOne<>(table, SQLResultAdapter.byClass(outputClass));
}
/**
* Fetch one
*
* @param table a query table context
* @param Type of jOOQ Table in Query context
* @return select one adapter
* @see TableLike
*/
static , R extends Record> SelectOne fetchOne(@NonNull T table) {
return new SelectOne<>(table, SQLResultAdapter.byTable(table));
}
/**
* Fetch one
*
* @param Type of jOOQ Table in Query context
* @param Type of record
* @param Type of expectation table
* @param table a query table context
* @return select one adapter
* @see TableLike
*/
static , R extends Record, Z extends Table> SelectOne fetchOne(
@NonNull T table, @NonNull Z toTable) {
return new SelectOne<>(table, SQLResultAdapter.byTable(toTable));
}
/**
* Fetch many Json record
*
* @param table a query table context
* @param Type of jOOQ Table in Query context
* @return select many adapter
* @see TableLike
* @see JsonRecord
*/
static > SelectList, JsonRecord>> fetchJsonRecords(
@NonNull T table) {
return new SelectList<>(table, SQLResultAdapter.byJson());
}
/**
* Fetch many
*
* @param table a query table context
* @param record record
* @param Type of jOOQ Table in Query context
* @param Type of record
* @return select many adapter
* @see TableLike
*/
static , R extends Record> SelectList fetchMany(@NonNull T table,
@NonNull R record) {
return new SelectList<>(table, SQLResultAdapter.byRecord(record));
}
/**
* Fetch many
*
* @param table a query table context
* @param fields given fields
* @param Type of jOOQ Table in Query context
* @return select many adapter
* @see TableLike
*/
static > SelectList fetchMany(@NonNull T table,
@NonNull Collection> fields) {
return new SelectList<>(table, SQLResultAdapter.byFields(fields));
}
/**
* Fetch many
*
* @param table a query table context
* @param outputClass given output class
* @param Type of jOOQ Table in Query context
* @param Type ot output class
* @return select many adapter
* @see TableLike
*/
static , R> SelectList, R> fetchMany(@NonNull T table,
@NonNull Class outputClass) {
return new SelectList<>(table, SQLResultAdapter.byClass(outputClass));
}
/**
* Fetch many
*
* @param table a query table context
* @param Type of jOOQ Table in Query context
* @return select many adapter
* @see TableLike
*/
static , R extends Record> SelectList fetchMany(@NonNull T table) {
return new SelectList<>(table, SQLResultAdapter.byTable(table));
}
/**
* Fetch many
*
* @param Type of jOOQ Table in Query context
* @param Type of record
* @param Type of expectation table
* @param table a query table context
* @return select many adapter
* @see TableLike
*/
static , R extends Record, Z extends Table> SelectList,
R> fetchMany(
@NonNull T table, @NonNull Z toTable) {
return new SelectList<>(table, SQLResultAdapter.byJson().andThen(r -> r.into(toTable)));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy