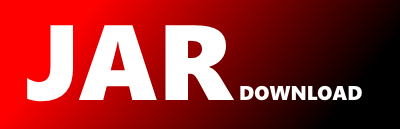
xxl.mathematica.network.Local Maven / Gradle / Ivy
package xxl.mathematica.network;
import io.vavr.control.Try;
import java.net.Inet4Address;
import java.net.Inet6Address;
import java.net.InetAddress;
import java.net.NetworkInterface;
import java.util.*;
import java.util.concurrent.Callable;
import java.util.function.Function;
/**
* 本机网络信息
*/
public class Local {
/**
* 所有网口信息
*
* @return
*/
public static List networkInterfaceNames() {
return Try.ofCallable(new Callable>() {
@Override
public List call() throws Exception {
List res = new ArrayList<>();
Enumeration interfaces = NetworkInterface.getNetworkInterfaces();
while (interfaces.hasMoreElements()) {
NetworkInterface e = interfaces.nextElement();
if (!e.isVirtual() && !e.isLoopback() && e.isUp()) {
res.add(e.getDisplayName());
}
}
return res;
}
}).get();
}
/**
* 所有网口信息
*
* @return
*/
public static List ipv4s() {
return Try.ofCallable(() -> {
List res = new ArrayList<>();
Enumeration interfaces = NetworkInterface.getNetworkInterfaces();
while (interfaces.hasMoreElements()) {
NetworkInterface e = interfaces.nextElement();
if (!e.isVirtual() && !e.isLoopback() && e.isUp()) {
Enumeration inetAddresses = e.getInetAddresses();
while (inetAddresses.hasMoreElements()) {
InetAddress inetAddress = inetAddresses.nextElement();
if (inetAddress instanceof Inet4Address) {
res.add(inetAddress.getHostAddress());
}
}
}
}
return res;
}).get();
}
/**
* 所有网口信息
*
* @return
*/
public static List ipv6s() {
return Try.ofCallable(() -> {
List res = new ArrayList<>();
Enumeration interfaces = NetworkInterface.getNetworkInterfaces();
while (interfaces.hasMoreElements()) {
NetworkInterface e = interfaces.nextElement();
if (!e.isVirtual() && !e.isLoopback() && e.isUp()) {
Enumeration inetAddresses = e.getInetAddresses();
while (inetAddresses.hasMoreElements()) {
InetAddress inetAddress = inetAddresses.nextElement();
if (inetAddress instanceof Inet6Address) {
res.add(inetAddress.getHostAddress());
}
}
}
}
return res;
}).get();
}
/**
* 所有网口信息
*
* @return
*/
public static List macs() {
return Try.ofCallable(() -> {
List res = new ArrayList<>();
Enumeration interfaces = NetworkInterface.getNetworkInterfaces();
while (interfaces.hasMoreElements()) {
NetworkInterface e = interfaces.nextElement();
byte[] mac = e.getHardwareAddress();
if (mac == null) {
continue;
}
res.add(io.vavr.collection.List.ofAll(mac)
.map(new Function() {
@Override
public String apply(Byte aByte) {
return String.format("%02X", aByte);
}
})
.mkString("-"));
}
return res;
}).get();
}
/**
* name mac ipv4 ipv6
*
* @return
*/
public static List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy