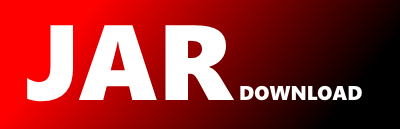
com.es.plus.adapter.core.EsPlusClient Maven / Gradle / Ivy
package com.es.plus.adapter.core;
import com.es.plus.adapter.params.EsAggResponse;
import com.es.plus.adapter.params.EsParamWrapper;
import com.es.plus.adapter.params.EsResponse;
import org.elasticsearch.action.bulk.BulkItemResponse;
import org.elasticsearch.client.RestHighLevelClient;
import org.elasticsearch.index.reindex.BulkByScrollResponse;
import java.time.Duration;
import java.util.Collection;
import java.util.List;
public interface EsPlusClient {
RestHighLevelClient getRestHighLevelClient();
/**
* 保存或更新批
*
* @param index 索引
* @param esDataList 西文数据列表
* @return {@link List}<{@link BulkItemResponse}>
*/
List saveOrUpdateBatch(String type, Collection> esDataList,String... index);
/**
* 保存批
*
* @param index 索引
* @param esDataList 西文数据列表
* @return {@link List}<{@link BulkItemResponse}>
*/
List saveBatch(String type, Collection> esDataList,String... index);
/**
* 保存
*/
boolean save(String type, Object esData,String... index);
/**
* 更新Es数据
*
* @param esData Es数据对象
* @return
* @throws Exception
*/
boolean update(String type, Object esData,String... index);
/**
* 批处理更新 返回失败数据
*
* @param index 索引
* @return {@link List}<{@link BulkItemResponse}>
*/
List updateBatch(String type, Collection> esDataList,String... index);
/**
* 更新包装
*/
BulkByScrollResponse updateByWrapper( String type, EsParamWrapper esUpdateWrapper,String... index);
/**
* 增量
*
* @param index 索引
* @param esUpdateWrapper es更新包装
* @return {@link BulkByScrollResponse}
*/
BulkByScrollResponse increment(String type, EsParamWrapper esUpdateWrapper,String... index);
/**
* 删除
*
* @param index 索引
* @param id id
* @return boolean
*/
boolean delete(String type, String id,String... index);
/**
* 删除,查询
*
* @param index 指数
* @param esUpdateWrapper es更新包装
* @return {@link BulkByScrollResponse}
*/
BulkByScrollResponse deleteByQuery(String type, EsParamWrapper esUpdateWrapper,String... index);
/**
* 删除批处理
*
* @param index 指数
* @param esDataList 西文数据列表
* @return boolean
*/
boolean deleteBatch(String type, Collection esDataList,String... index);
/**
* 统计数
*
* @param esParamWrapper es查询包装
* @param index 指数
* @return long
*/
long count(String type, EsParamWrapper esParamWrapper,String... index);
/**
* 搜索包装
*
* @param esParamWrapper es查询包装
* @param index 指数
* @return {@link EsResponse}<{@link T}>
*/
EsResponse search(String type, EsParamWrapper esParamWrapper,String... index);
/**
* 滚动查询包装
*
* @param esParamWrapper es查询包装
* @param index 指数
* @param keepTime 保持时间
* @return
*/
EsResponse scroll(String type, EsParamWrapper esParamWrapper, Duration keepTime, String scrollId,String... index);
/**
* 聚合
*
* @param index 指数
* @param esParamWrapper es查询包装
*/
EsAggResponse aggregations(String type, EsParamWrapper esParamWrapper,String... index);
/**
* 执行dsl
*
* @param dsl dsl
* @param index 索引名字
* @return {@link String}
*/
String executeDSL(String dsl, String... index);
/**
* 翻译sql
*
* @return {@link String}
*/
String translateSql(String sql);
/**
* 执行sql
*
* @return {@link String}
*/
EsResponse executeSQL(String sql,Class tClass);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy