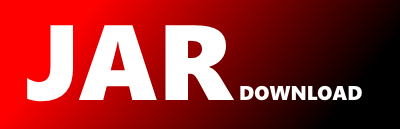
com.es.plus.adapter.util.BeanUtils Maven / Gradle / Ivy
package com.es.plus.adapter.util;
import org.springframework.cglib.beans.BeanMap;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class BeanUtils {
public static Map beanToMap(T bean) {
BeanMap beanMap = BeanMap.create(bean);
Map map = new HashMap<>();
beanMap.forEach((key, value) -> {
map.put(String.valueOf(key), value);
});
return map;
}
public static Map beanToMapIgnoreNull(T bean) {
BeanMap beanMap = BeanMap.create(bean);
Map map = new HashMap<>();
beanMap.forEach((key, value) -> {
if (value != null) {
map.put(String.valueOf(key), value);
}
});
return map;
}
public static T mapToBean(Map map, Class clazz) {
if (clazz.equals(Map.class)) {
return (T) map;
}
T bean = null;
try {
bean = clazz.newInstance();
} catch (InstantiationException | IllegalAccessException e) {
throw new RuntimeException("instance error mapToBean");
}
BeanMap beanMap = BeanMap.create(bean);
beanMap.putAll(map);
return bean;
}
public static List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy