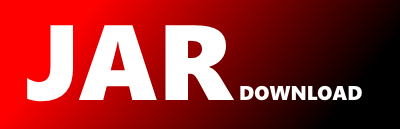
com.cloud.platform.web.utils.CommonUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cloud-web-spring-boot-starter Show documentation
Show all versions of cloud-web-spring-boot-starter Show documentation
project for cloud-web-spring-boot-starter
The newest version!
package com.cloud.platform.web.utils;
import com.cloud.platform.common.constants.PlatformCommonConstant;
import com.cloud.platform.common.utils.JsonUtil;
import com.google.common.base.Joiner;
import com.google.common.base.Splitter;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.collections.CollectionUtils;
import org.apache.commons.collections.MapUtils;
import org.apache.commons.lang3.StringUtils;
import org.springframework.cglib.beans.BeanMap;
import org.springframework.objenesis.instantiator.util.ClassUtils;
import java.util.*;
import java.util.function.Consumer;
import java.util.stream.Collectors;
@Slf4j
public class CommonUtil {
private CommonUtil() {
}
/**
* 判断字符串不为空
*
* @param str
* @return
*/
public static boolean isNotEmpty(String str) {
return StringUtils.isNotBlank(str);
}
/**
* 判断字符串为空
*
* @param str
* @return
*/
public static boolean isEmpty(String str) {
return StringUtils.isBlank(str);
}
/**
* 判断集合不为空
*
* @param collection
* @return
*/
public static boolean isNotEmpty(Collection collection) {
return CollectionUtils.isNotEmpty(collection);
}
/**
* 判断集合为空
*
* @param collection
* @return
*/
public static boolean isEmpty(Collection collection) {
return CollectionUtils.isEmpty(collection);
}
/**
* 判断Map不为空
*
* @param map
* @return
*/
public static boolean isNotEmpty(Map map) {
return MapUtils.isNotEmpty(map);
}
/**
* 判断Map为空
*
* @param map
* @return
*/
public static boolean isEmpty(Map map) {
return MapUtils.isEmpty(map);
}
/**
* 对象字符串转换为List集合
*/
public static List strToList(String str, Class clazz) {
return JsonUtil.toList(str);
}
/**
* 对象字符串转换为对象
*/
public static T strToBean(String str, Class clazz) {
return JsonUtil.toBean(str, clazz);
}
/**
* 对象转Map
*
* @param bean
* @return
*/
public static Map beanToMap(Object bean) {
return Objects.isNull(bean) ? null : BeanMap.create(bean);
}
/**
* map转对象
*
* @param map
* @param clazz
* @param
* @return
*/
public static T mapToBean(Map map, Class clazz) {
T bean = ClassUtils.newInstance(clazz);
BeanMap.create(bean).putAll(map);
return bean;
}
/**
* List 转 list
© 2015 - 2024 Weber Informatics LLC | Privacy Policy