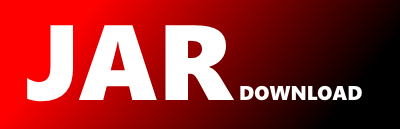
com.cloud.platform.common.utils.JsonUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of platform-common Show documentation
Show all versions of platform-common Show documentation
project for platform-common
The newest version!
package com.cloud.platform.common.utils;
import com.cloud.platform.common.serializer.LongToStringSerializer;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.DeserializationFeature;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.SerializationFeature;
import com.fasterxml.jackson.datatype.jsr310.JavaTimeModule;
import com.fasterxml.jackson.datatype.jsr310.deser.LocalDateDeserializer;
import com.fasterxml.jackson.datatype.jsr310.deser.LocalDateTimeDeserializer;
import com.fasterxml.jackson.datatype.jsr310.ser.LocalDateSerializer;
import com.fasterxml.jackson.datatype.jsr310.ser.LocalDateTimeSerializer;
import lombok.extern.slf4j.Slf4j;
import java.text.SimpleDateFormat;
import java.time.LocalDate;
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
import java.util.List;
import java.util.Map;
/**
* @Description:
* @Author: ZhouShuai
* @Date: 2021-06-27 17:04
*/
@Slf4j
public class JsonUtil {
public static final ObjectMapper OBJECT_MAPPER = new ObjectMapper();
private static final SimpleDateFormat DATE_FORMAT_TIME = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
private static final DateTimeFormatter MY_DATE_TIME = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss");
private static final DateTimeFormatter MY_DATE = DateTimeFormatter.ofPattern("yyyy-MM-dd");
private JsonUtil() {
}
private static String writeValue(Object object) {
if (object == null) {
return null;
} else if (object instanceof String) {
return (String)object;
} else {
try {
return OBJECT_MAPPER.writeValueAsString(object);
} catch (JsonProcessingException var2) {
log.error("writeJsonValue error, ", var2);
return null;
}
}
}
private static T readValue(String json, Class t) {
if (json == null) {
return null;
} else {
try {
return OBJECT_MAPPER.readValue(json, t);
} catch (Exception var3) {
log.error("readJsonValue error, ", var3);
return null;
}
}
}
private static T readValue(String json, TypeReference t) {
if (json == null) {
return null;
} else {
try {
return OBJECT_MAPPER.readValue(json, t);
} catch (Exception var3) {
log.error("readJsonValue error, ", var3);
return null;
}
}
}
public static String toString(Object object) {
return writeValue(object);
}
public static T toBean(String json, Class t) {
return readValue(json, t);
}
public static List toList(String json) {
return (List)readValue(json, new TypeReference>() {
});
}
public static Map toMap(String json) {
return (Map)readValue(json, new TypeReference
© 2015 - 2024 Weber Informatics LLC | Privacy Policy