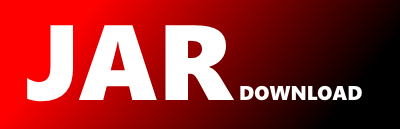
commonMain.ru.casperix.math.array.int32.IntMap2D.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of math-jvm Show documentation
Show all versions of math-jvm Show documentation
Simple set of geometric and other types
package ru.casperix.math.array.int32
import ru.casperix.math.array.ArrayAccessND
import ru.casperix.math.array.int8.ByteMap2D
import ru.casperix.math.array.IndexedMap2D
import ru.casperix.math.array.MutableMap2D
import ru.casperix.math.axis_aligned.int32.Box2i
import ru.casperix.math.vector.int32.Vector2i
import kotlinx.serialization.Serializable
@Serializable
data class IntMap2D(override val dimension: Vector2i, val array: IntArray) : IndexedMap2D, MutableMap2D {
override fun setByIndex(index: Int, value: Int) {
array[index] = value
}
override fun getByIndex(index: Int): Int {
return array[index]
}
companion object {
fun createByXY(dimension: Vector2i, builder: (position: Vector2i) -> Int): IntMap2D {
val items = IntArray(dimension.volume())
for (y in 0 until dimension.y) {
for (x in 0 until dimension.x) {
val position = Vector2i(x, y)
val index = ArrayAccessND.index2D(dimension, position)
items.set(index, builder(position))
}
}
return IntMap2D(dimension, items)
}
fun create(dimension: Vector2i, builder: (index: Int) -> Int): IntMap2D {
return IntMap2D(dimension, IntArray(dimension.volume()) { builder(it) })
}
fun unionChannel(
dimension: Vector2i,
getRed: (Int) -> Byte,
getGreen: (Int) -> Byte,
getBlue: (Int) -> Byte,
getAlpha: (Int) -> Byte
): IntMap2D {
TODO()
// val bytes = IntArray(dimension.x * dimension.y) { ColorDecoder.bytesToColor(getRed(it), getGreen(it), getBlue(it), getAlpha(it)) }
// val map = IntMap2D(dimension, bytes)
// return map
}
}
init {
if (array.size != dimension.volume()) throw Error("Invalid array size. Need: ${dimension.volume()}")
}
override fun equals(other: Any?): Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as IntMap2D
if (!array.contentEquals(other.array)) return false
if (dimension != other.dimension) return false
return true
}
override fun hashCode(): Int {
var result = array.contentHashCode()
result = 31 * result + dimension.hashCode()
return result
}
fun takeRegion(region: Box2i): IntMap2D {
val array = IntArray(region.volume) { targetIndex ->
val targetPosition = ArrayAccessND.position2D(region.dimension, targetIndex)
get(targetPosition + region.min)
}
return IntMap2D(region.dimension, array)
}
fun rotate180CW(): IntMap2D {
return rotate90CW().rotate90CW()
}
fun rotate180CCW(): IntMap2D {
return rotate90CCW().rotate90CCW()
}
/**
* Поворот на 90 градусов по часовой стрелке
*/
fun rotate90CW(): IntMap2D {
val dimension = Vector2i(sizeY, sizeX)
val array = IntArray(array.size) { index ->
val pos = position2D(index)
get(pos.y, dimension.y - 1 - pos.x)
}
return IntMap2D(dimension, array)
}
/**
* Поворот на 90 градусов против часовой стрелки
*/
fun rotate90CCW(): IntMap2D {
val dimension = Vector2i(sizeY, sizeX)
val array = IntArray(array.size) { index ->
val pos = position2D(index)
get(dimension.x - 1 - pos.y, pos.x)
}
return IntMap2D(dimension, array)
}
fun flipX(source: IntMap2D): IntMap2D {
val dimension = dimension
val array = IntArray(array.size) { index ->
val pos = position2D(index)
get(dimension.x - 1 - pos.x, pos.y)
}
return IntMap2D(dimension, array)
}
fun flipY(): IntMap2D {
val dimension = dimension
val array = IntArray(array.size) { index ->
val pos = position2D(index)
get(pos.x, dimension.y - 1 - pos.y)
}
return IntMap2D(dimension, array)
}
private fun position2D(index: Int): Vector2i {
return ArrayAccessND.position2D(dimension, index)
}
fun takeChannel(channelIndex: Int): ByteMap2D {
TODO()
// val bytes = ByteArray(array.size) { ColorDecoder.decodeComponentAsByte(channelIndex, array[it]) }
// return ByteMap2D(dimension, bytes)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy