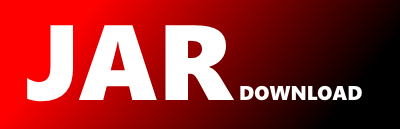
commonMain.ru.casperix.math.curve.float64.BezierQuadratic2d.kt Maven / Gradle / Ivy
package ru.casperix.math.curve.float64
import ru.casperix.math.curve.CurveHelper
import ru.casperix.math.interpolation.float64.InterpolationDouble
import ru.casperix.math.vector.float64.Vector2d
import kotlinx.serialization.Serializable
import kotlin.math.pow
@Serializable
data class BezierQuadratic2d(val p0: Vector2d, val p1: Vector2d, val p2: Vector2d) : LazyCurve2d() {
override fun getPosition(t: Double): Vector2d {
val s = 1f - t
val k0 = s.pow(2)
val k1 = 2f * s * t
val k2 = t.pow(2)
return Vector2d(
p0.x * k0 + p1.x * k1 + p2.x * k2,
p0.y * k0 + p1.y * k1 + p2.y * k2,
)
}
override fun length(): Double {
return CurveHelper.calculateLength(this, 10)
}
override fun divide(t: Double): Pair {
val AB = InterpolationDouble.vector2(p0, p1, t)
val BC = InterpolationDouble.vector2(p1, p2, t)
val H = InterpolationDouble.vector2(AB, BC, t)
return Pair(BezierQuadratic2d(p0, AB, H), BezierQuadratic2d(H, BC, p2))
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy