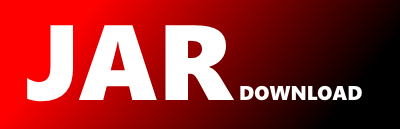
commonMain.ru.casperix.math.iteration.MapIterator.kt Maven / Gradle / Ivy
package ru.casperix.math.iteration
fun Iterator.firstOrNull(condition: (T) -> Boolean): T? {
forEach {
if (condition(it)) return it
}
return null
}
fun Iterator.firstOrNull(): T? {
forEach {
return it
}
return null
}
fun MutableIterator.removeIf(action: (T) -> Boolean) {
forEach {
if (action(it)) {
remove()
}
}
}
fun Iterator.map(converter: (Source) -> Target?): Iterator {
return MapIterator(this, converter)
}
class MapIterator(val sourceItems: Iterator, val map: (source: Source) -> Target?) : Iterator {
private var current: Target? = null
init {
current = getNext()
}
private fun getNext(): Target? {
while (sourceItems.hasNext()) {
val pos = sourceItems.next()
map(pos)?.let {
return it
}
}
return null
}
override fun next(): Target {
val last = current ?: throw Error("Invalid next. You must check hasNext first")
current = getNext()
return last
}
override fun hasNext(): Boolean {
return current != null
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy