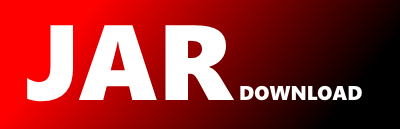
commonMain.ru.casperix.math.vector.int32.Vector3i.kt Maven / Gradle / Ivy
package ru.casperix.math.vector.int32
import ru.casperix.math.axis_aligned.int32.Box3i
import ru.casperix.math.axis_aligned.int32.Dimension3i
import ru.casperix.math.vector.api.AbstractVector3
import ru.casperix.math.vector.float32.Vector3f
import ru.casperix.math.vector.float64.Vector3d
import ru.casperix.math.vector.uint32.Vector3u
import ru.casperix.misc.format.FormatType
import ru.casperix.misc.toPrecision
import kotlinx.serialization.Serializable
import kotlin.math.*
@Serializable
data class Vector3i(override val x: Int, override val y: Int, override val z: Int) : AbstractVector3 {
constructor() : this(0)
constructor(i: Int) : this(i, i, i)
companion object {
val ZERO = Vector3i(0)
val ONE = Vector3i(1)
val XYZ = ONE
val X = Vector3i(1, 0, 0)
val Y = Vector3i(0, 1, 0)
val Z = Vector3i(0, 0, 1)
val YZ = Vector3i(0, 1, 1)
val XZ = Vector3i(1, 0, 1)
val XY = Vector3i(1, 1, 0)
}
override val xAxis: Vector3i get() = Vector3i(x, 0, 0)
override val yAxis: Vector3i get() = Vector3i(0, y, 0)
override val zAxis: Vector3i get() = Vector3i(0, 0, z)
override fun volume(): Int {
return x * y * z
}
override fun distTo(other: Vector3i): Int {
return (this - other).length()
}
override fun lengthOne(): Int {
return abs(x) + abs(y) + abs(z)
}
override fun length(): Int {
return sqrt((x * x + y * y + z * z).toFloat()).roundToInt()
}
override fun lengthInf(): Int {
return maxOf(abs(x), abs(y), abs(z))
}
override fun lengthSquared(): Int {
return x * x + y * y + z * z
}
override fun absoluteMinimum(): Int {
return minOf(abs(x), abs(y), abs(z))
}
override fun absoluteMaximum(): Int {
return maxOf(abs(x), abs(y), abs(z))
}
override val sign: Vector3i get() = Vector3i(x.sign, y.sign, z.sign)
override val absoluteValue: Vector3i get() = Vector3i(x.absoluteValue, y.absoluteValue, z.absoluteValue)
override fun dot(value: Vector3i): Int {
return (this.x * value.x + this.y * value.y + this.z * value.z)
}
override fun mod(other: Vector3i): Vector3i {
return Vector3i(x.mod(other.x), y.mod(other.y), z.mod(other.z))
}
override fun upper(other: Vector3i): Vector3i {
return Vector3i(max(x, other.x), max(y, other.y), max(z, other.z))
}
override fun lower(other: Vector3i): Vector3i {
return Vector3i(min(x, other.x), min(y, other.y), min(z, other.z))
}
fun clamp(min: Vector3i, max: Vector3i): Vector3i {
return upper(min).lower(max)
}
fun clamp(area: Box3i): Vector3i {
return clamp(area.min, area.max)
}
override operator fun plus(position: Vector3i): Vector3i {
return Vector3i(x + position.x, y + position.y, z + position.z)
}
override operator fun minus(position: Vector3i): Vector3i {
return Vector3i(x - position.x, y - position.y, z - position.z)
}
override operator fun div(value: Int): Vector3i {
return Vector3i(x / value, y / value, z / value)
}
override operator fun div(value: Vector3i): Vector3i {
return Vector3i(x / value.x, y / value.y, z / value.z)
}
override operator fun times(value: Int): Vector3i {
return Vector3i(x * value, y * value, z * value)
}
override operator fun times(value: Vector3i): Vector3i {
return Vector3i(x * value.x, y * value.y, z * value.z)
}
override operator fun unaryMinus(): Vector3i {
return Vector3i(-x, -y, -z)
}
override operator fun rem(value: Vector3i): Vector3i {
return Vector3i(x % value.x, y % value.y, z % value.z)
}
override operator fun rem(value: Int): Vector3i {
return Vector3i(x % value, y % value, z % value)
}
override fun greater(other: Vector3i): Boolean {
return x > other.x && y > other.y && z > other.z
}
override fun greaterOrEq(other: Vector3i): Boolean {
return x >= other.x && y >= other.y && z >= other.z
}
override fun less(other: Vector3i): Boolean {
return x < other.x && y < other.y && z < other.z
}
override fun lessOrEq(other: Vector3i): Boolean {
return x <= other.x && y <= other.y && z <= other.z
}
// fun addDimension(w: Float): Vector4i {
// return Vector4i(x, y, z, w)
// }
override fun toVector3f(): Vector3f {
return Vector3f(x.toFloat(), y.toFloat(), z.toFloat())
}
override fun toVector3d(): Vector3d {
return Vector3d(x.toDouble(), y.toDouble(), z.toDouble())
}
override fun toVector3i(): Vector3i {
return this
}
override fun toVector3u(): Vector3u {
return Vector3u(x.toUInt(), y.toUInt(), z.toUInt())
}
fun toDimension3i(): Dimension3i {
return Dimension3i(x, y, z)
}
override fun normalize(): Vector3i {
return toVector3f().normalize().roundToVector3i()
}
fun getXY(): Vector2i {
return Vector2i(x, y)
}
fun getYZ(): Vector2i {
return Vector2i(y, z)
}
fun getXZ(): Vector2i {
return Vector2i(x, z)
}
fun expand(w: Int): Vector4i {
return Vector4i(x, y, z, w)
}
override fun half(): Vector3i {
return this / 2
}
override fun toString(): String {
return format()
}
fun format(type: FormatType = FormatType.NORMAL): String {
return when (type) {
FormatType.DETAIL -> "Vector3i(x=$x, y=$y, z=$z)"
FormatType.NORMAL -> "V3i($x, $y, $z)"
FormatType.SHORT -> "(${x},${y},${z})"
}
}
fun component(index: Int): Int {
return when (index) {
0 -> x
1 -> y
2 -> z
else -> throw Error("Only 3 components enabled")
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy