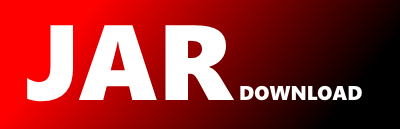
commonMain.ru.casperix.signals.collection.ObservableMutableMap.kt Maven / Gradle / Ivy
package ru.casperix.signals.collection
import ru.casperix.signals.concrete.Future
import ru.casperix.signals.concrete.Signal
class ObservableMutableMap : MutableMap, ObservableMap {
private val map = mutableMapOf()
private val addSignal = Signal>()
private val removeSignal = Signal>()
override fun addFuture(): Future> {
return addSignal
}
override fun removeFuture(): Future> {
return removeSignal
}
override fun get(key: Key): Value? {
return map[key]
}
override fun remove(key: Key): Value? {
val element = map.remove(key) ?: return null
removeSignal.set(object : Map.Entry {
override val key = key
override val value = element
})
return element
}
override val entries: MutableSet>
get() = map.entries
override val keys: MutableSet
get() = map.keys
override val values: MutableCollection
get() = map.values
override fun containsKey(key: Key): Boolean {
return map.containsKey(key)
}
override fun containsValue(value: Value): Boolean {
return map.containsValue(value)
}
override fun isEmpty(): Boolean {
return map.isEmpty()
}
override val size: Int
get() = map.size
override fun clear() {
entries.forEach {
removeSignal.set(it)
}
map.clear()
}
override fun put(key: Key, value: Value): Value? {
if (map.containsKey(key)) return null
map[key] = value
addSignal.set(object : Map.Entry {
override val key = key
override val value = value
})
return value
}
override fun putAll(from: Map) {
from.forEach {
put(it.key, it.value)
}
}
// override fun contains(element: Map.Entry): Boolean {
// return map.get(element.key) == element.value
// }
//
// override fun containsAll(elements: Collection>): Boolean {
// elements.forEach {
// if (!contains(it)) return false
// }
// return true
// }
// override fun iterator(): Iterator> {
// return map.iterator()
// }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy