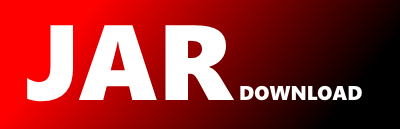
commonMain.ru.casperix.spine.json.CurveSerializer.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spine Show documentation
Show all versions of spine Show documentation
Signals for all occasions
package ru.casperix.spine.json
import ru.casperix.math.vector.float32.Vector2f
import kotlinx.serialization.KSerializer
import kotlinx.serialization.descriptors.SerialDescriptor
import kotlinx.serialization.descriptors.buildClassSerialDescriptor
import kotlinx.serialization.encoding.Decoder
import kotlinx.serialization.encoding.Encoder
import kotlinx.serialization.json.*
import ru.casperix.spine.json.component.BezierCurve
import ru.casperix.spine.json.component.Curve
import ru.casperix.spine.json.component.SteppedCurve
import ru.casperix.spine.json.component.TupleBezierCurve
class CurveSerializer : KSerializer {
override val descriptor: SerialDescriptor = buildClassSerialDescriptor("Curve")
override fun deserialize(decoder: Decoder): Curve {
require(decoder is JsonDecoder)
when (val element = decoder.decodeJsonElement()) {
is JsonArray -> {
val floats = element.jsonArray.map { it.jsonPrimitive.content.toFloat() }
if (floats.size == 4) {
return BezierCurve(Vector2f(floats[0], floats[1]), Vector2f(floats[2], floats[3]))
} else if (floats.size == 8) {
return TupleBezierCurve(
BezierCurve(Vector2f(floats[0], floats[1]), Vector2f(floats[2], floats[3])),
BezierCurve(Vector2f(floats[4], floats[5]), Vector2f(floats[6], floats[7]))
)
}
throw Exception("Expected 4 or 8 points. Actual: ${floats.size}")
}
is JsonPrimitive -> {
val content = element.content
if (content == "stepped") {
return SteppedCurve
} else {
throw Exception("Unsupported or invalid format")
}
}
else -> {
throw Exception("Unsupported or invalid format")
}
}
}
override fun serialize(encoder: Encoder, value: Curve) {
TODO("Not yet implemented")
}
}
//object CurveSerializer : JsonContentPolymorphicSerializer(Curve::class) {
// override fun selectDeserializer(element: JsonElement) = when {
// element is JsonArray -> QuadraticCurve(element.map { it.jsonPrimitive.content.toFloat() }).serializer()
// element is JsonPrimitive -> if (element.content == "stepped") {
// SteppedCurve.serializer()
// } else {
// throw Exception("Unsupported or invalid format")
// }
//
// else -> throw Exception("Unsupported or invalid format")
// }
//}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy