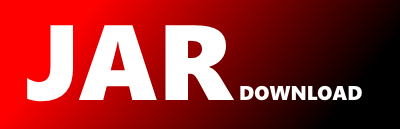
commonMain.ru.casperix.spine.binary.BinarySkeletonBuffer.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spine Show documentation
Show all versions of spine Show documentation
Signals for all occasions
package ru.casperix.spine.binary
import ru.casperix.math.color.Color
import ru.casperix.math.color.ColorCode
class BinarySkeletonBuffer(val source: UByteArray) {
private var readIndex = 0
fun readByte(): Int {
return source[readIndex++].toInt()
}
fun readByteToLong(): Long {
return source[readIndex++].toLong()
}
fun readShort(): Int {
return readByte() shl 8 or readByte()
}
fun readInt(): Int {
return readByte() shl 24 or (readByte() shl 16) or (readByte() shl 8) or readByte()
}
fun readBytes(length: Int): UByteArray {
readIndex += length
return source.slice(readIndex - length until readIndex).toUByteArray()
}
fun readLong(): Long {
return readByteToLong() shl 56 or readByteToLong() shl 48 or readByteToLong() shl 40 or readByteToLong() shl 32 or readByteToLong() shl 24 or (readByteToLong() shl 16) or (readByteToLong() shl 8) or readByteToLong()
}
fun readVarint(optimizePositive: Boolean = false): Long {
var byte: Int
var value: Long = 0
var shift = 0
do {
byte = readByte()
value = value or (byte.toLong() and 0x7FL shl shift)
shift += 7
} while (byte and 0x80 != 0)
if (!optimizePositive) {
value = ((value shr 1) xor (-(value and 1L)))
}
return value
}
// fun readVarint(optimizePositive: Boolean = false): Long {
// var b = readByte()
// var value: Long = (b and 0x7F).toLong()
// if (b and 0x80 != 0) {
// b = readByte();
// value = value or (b and 0x7F).toLong() shl 7
// if (b and 0x80 != 0) {
// b = readByte();
// value = value or (b and 0x7F).toLong() shl 14
// if (b and 0x80 != 0) {
// b = readByte();
// value = value or (b and 0x7F).toLong() shl 21
// if (b and 0x80 != 0) value = value or (readByte() and 0x7F).toLong() shl 28
// }
// }
// }
// if (!optimizePositive) value = ((value shr 1) xor (-(value and 1L)))
// return value
// }
fun readFloat(): Float {
return Float.fromBits(readInt())
}
fun readString(maxLength: Int = Int.MAX_VALUE): String? {
var count = readVarint(true).toInt()
if (count == 0) {
return null
}
if (count-- > 1L) {
if (count >= maxLength) count = maxLength - 1
val result = source.asByteArray().decodeToString(readIndex, readIndex + count)
readIndex += count
return result
}
return ""
}
fun readRefString(): Int? {
val index = readVarint(true).toInt()
if (index == 0) {
return null
} else {
return index - 1
}
}
fun readColor(): Color {
return ColorCode(readInt().toUInt())
}
fun readBoolean(): Boolean {
return readByte().run {
if (this == 0) return@run false
if (this == 1) return@run true
throw Exception("Invalid boolean for index: ${readIndex - 1}")
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy