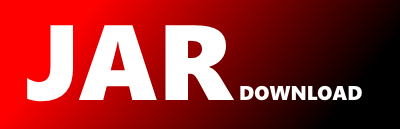
ch.openchvote.algorithms.plain.CheckDecryptionProof Maven / Gradle / Ivy
/*
* Copyright (C) 2023 Berner Fachhochschule https://e-voting.bfh.ch
*
* - This program is free software: you can redistribute it and/or modify -
* - it under the terms of the GNU Affero General Public License as published by -
* - the Free Software Foundation, either version 3 of the License, or -
* - (at your option) any later version. -
* - -
* - This program is distributed in the hope that it will be useful, -
* - but WITHOUT ANY WARRANTY; without even the implied warranty of -
* - MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the -
* - GNU General Public License for more details. -
* - -
* - You should have received a copy of the GNU Affero General Public License -
* - along with this program. If not, see . -
*/
package ch.openchvote.algorithms.plain;
import ch.openchvote.algorithms.Precondition;
import ch.openchvote.algorithms.general.GetChallenge;
import ch.openchvote.model.common.Encryption;
import ch.openchvote.model.plain.DecryptionProof;
import ch.openchvote.parameters.security.NIZKPParameters;
import ch.openchvote.parameters.security.ZZPlusParameters;
import ch.openchvote.util.sequence.Vector;
import ch.openchvote.util.set.Set;
import ch.openchvote.util.algebra.ZZPlus;
import ch.openchvote.util.algebra.ZZ;
import ch.openchvote.util.tools.Parallel;
import ch.openchvote.util.tuples.Triple;
import java.math.BigInteger;
/**
* ALGORITHM 8.51
*/
public class CheckDecryptionProof {
public static
boolean run(DecryptionProof pi, BigInteger pk, Vector bold_e, Vector bold_c, P params) {
// PARAMETERS
Precondition.checkNotNull(params);
var p = params.get_p();
var q = params.get_q();
var g = params.get_g();
var ZZPlus_p = ZZPlus.of(p);
var ZZ_q = ZZ.of(q);
var tau = params.get_tau();
var ZZ_twoToTheTau = ZZ.ofExp(tau);
// PREPARATION
Precondition.checkNotNull(pi, pk, bold_e, bold_c);
var N = bold_e.getLength();
Precondition.check(Set.Pair(ZZ_twoToTheTau, ZZ_q).contains(pi));
Precondition.check(ZZPlus_p.contains(pk));
Precondition.check(Set.Vector(Set.Pair(ZZPlus_p, ZZPlus_p), N).contains(bold_e));
Precondition.check(Set.Vector(ZZPlus_p, N).contains(bold_c));
var c = pi.get_c();
var s = pi.get_s();
var builder_bold_t = new Vector.Builder(0, N);
// ALGORITHM
var t_0 = ZZPlus_p.multiply(ZZPlus_p.pow(pk, c), ZZPlus_p.pow(g, s));
builder_bold_t.setValue(0, t_0);
Parallel.forLoop(1, N, i -> {
var c_i = bold_c.getValue(i);
var b_i = bold_e.getValue(i).get_b();
var t_i = ZZPlus_p.multiply(ZZPlus_p.pow(c_i, c), ZZPlus_p.pow(b_i, s));
builder_bold_t.setValue(i, t_i);
});
var bold_t = builder_bold_t.build();
var y = new Triple<>(pk, bold_e, bold_c);
var c_prime = GetChallenge.run(y, bold_t, params);
return c.equals(c_prime);
}
}