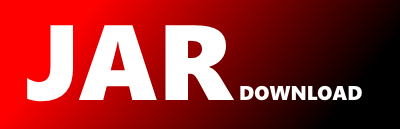
ch.openchvote.framework.services.MessagingService Maven / Gradle / Ivy
/*
* Copyright (C) 2024 Berner Fachhochschule https://e-voting.bfh.ch
*
* - This program is free software: you can redistribute it and/or modify -
* - it under the terms of the GNU Affero General Public License as published by -
* - the Free Software Foundation, either version 3 of the License, or -
* - (at your option) any later version. -
* - -
* - This program is distributed in the hope that it will be useful, -
* - but WITHOUT ANY WARRANTY; without even the implied warranty of -
* - MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the -
* - GNU General Public License for more details. -
* - -
* - You should have received a copy of the GNU Affero General Public License -
* - along with this program. If not, see . -
*/
package ch.openchvote.framework.services;
import ch.openchvote.framework.communication.Message;
/**
* A messaging service can be used by the parties participating in a protocol to exchange signed messages with other
* protocol participants. The interface provides methods for subscribing a party to the messaging service, unsubscribing
* a party from the messaging service, and for sending a signed {@link Message} from one subscribed party to another
* subscribed party. Upon receiving a signed message, the receiver's method
* {@link MessagingService.Subscriber#onMessage(Message)} is called.
*/
public interface MessagingService {
/**
* This sub-interface defines the sender's part of the messaging service needed for sending messages.
*/
interface Source extends Service {
/**
* Sends the given signed message to the intended receiver.
*
* @param message The given signed message
*/
void send(Message message);
}
/**
* This sub-interface defines the subscriber's part of the messaging service. It provides methods for subscribing
* and unsubscribing.
*/
interface Target extends Service {
/**
* Subscribes the given subscriber to the messaging service. Nothing happens if the subscriber is already
* subscribed.
*
* @param subscriber The given subscriber
*/
void subscribe(MessagingService.Subscriber subscriber);
/**
* Unsubscribes the given subscriber from the messaging service. Nothing happens if currently the subscriber is
* not subscribed to the messaging service.
*
* @param subscriber The given subscriber
*/
void unsubscribe(MessagingService.Subscriber subscriber);
}
/**
* This sub-interface defines the method that a messaging service subscriber must provide for receiving signed
* messages.
*/
interface Subscriber extends Service.Subscriber {
/**
* This method is called upon receiving an incoming signed message.
*
* @param message The received signed message
*/
void onMessage(Message message);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy