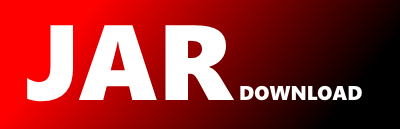
io.gitlab.schedule4j.cron.subpart.AllNumbersSubpart Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of schedule4j-cron Show documentation
Show all versions of schedule4j-cron Show documentation
Library for parsing cron expressions and calculate time points from cron expressions
The newest version!
/*
* Created on 2010-03-01
*
* Copyright 2010 Dirk Buchhorn
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.gitlab.schedule4j.cron.subpart;
import io.gitlab.schedule4j.cron.CronResult;
import java.time.temporal.ChronoField;
/**
* This class represents the '*' in a cron part.
*
* @author Dirk Buchhorn
*/
public class AllNumbersSubpart extends AbstractCalendarFieldSubpart
{
public AllNumbersSubpart(ChronoField chronoField)
{
super(chronoField);
}
@Override
public boolean check(int value, int minValue, int maxValue)
{
boolean check = false;
if (minValue <= value && value <= maxValue)
{
check = true;
}
return check;
}
@Override
public CronResult getNext(int value, int minValue, int maxValue)
{
CronResult cronResult = new CronResult();
int next = value + 1;
next = Math.max(next, minValue);
// this can't occur, because it's only for YEAR (!rotating) and maxValue is Integer.MAX_VALUE
// if (next > maxValue && !isRotatingNumbers())
// {
// return null;
// }
if (next > maxValue)
{
next = minValue;
cronResult.setAddCarry(1);
}
cronResult.setNumber(next);
return cronResult;
}
@Override
public CronResult getPrevious(int value, int minValue, int maxValue)
{
CronResult cronResult = new CronResult();
int previous = value - 1;
previous = Math.min(previous, maxValue);
// this can't occur, because it's only for YEAR (!rotating) and minValue is Integer.MIN_VALUE
// if (previous < minValue && !isRotatingNumbers())
// {
// return null;
// }
if (previous < minValue)
{
previous = maxValue;
cronResult.setAddCarry(-1);
}
cronResult.setNumber(previous);
return cronResult;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy