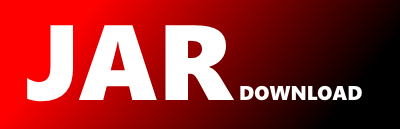
io.gitlab.schedule4j.cron.subpart.RangeNumbersSubpart Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of schedule4j-cron Show documentation
Show all versions of schedule4j-cron Show documentation
Library for parsing cron expressions and calculate time points from cron expressions
The newest version!
/*
* Created on 2010-02-20
*
* Copyright 2010 Dirk Buchhorn
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.gitlab.schedule4j.cron.subpart;
import io.gitlab.schedule4j.cron.CronResult;
import java.time.temporal.ChronoField;
/**
* This class represents a number range (like 2-13, 0/5, 10-30/2) in a cron part.
*
* @author Dirk Buchhorn
*/
public class RangeNumbersSubpart extends NumbersSubpart
{
private int startValue;
private int endValue;
private int increment;
public RangeNumbersSubpart(ChronoField chronoField, int startValue, int endValue, int increment)
{
super(chronoField, startValue);
if (chronoField == ChronoField.YEAR && startValue > endValue)
{
throw new IllegalArgumentException("startValue can't be greater than the endValue for a year field");
}
this.startValue = startValue;
this.endValue = endValue;
this.increment = increment;
if (chronoField != ChronoField.YEAR)
{
calculateAllowedValues();
}
}
private void calculateAllowedValues()
{
int minValue = (int) getChronoField().range().getMinimum();
int maxValue = (int) getChronoField().range().getMaximum();
// some ranges start with 0 and some with 1
int correctedMaxValue = maxValue + 1 - minValue;
int end = startValue <= endValue ? endValue : (correctedMaxValue + endValue);
int value = startValue;
// start value is already added
while ((value += increment) <= end)
{
int number = value > maxValue ? value - correctedMaxValue : value;
if (number >= minValue)
{
addNumber(number);
}
}
}
public int getStartValue()
{
return startValue;
}
public int getEndValue()
{
return endValue;
}
public int getIncrement()
{
return increment;
}
@Override
public boolean check(int value, int minValue, int maxValue)
{
boolean accepted = false;
if (getChronoField() == ChronoField.YEAR)
{
if (value >= startValue && value <= endValue)
{
int mod = (value - startValue) % increment;
accepted = mod == 0;
}
}
else
{
accepted = super.check(value, minValue, maxValue);
}
return accepted;
}
@Override
public CronResult getNext(int value, int minValue, int maxValue)
{
CronResult cronResult = null;
if (getChronoField() == ChronoField.YEAR)
{
if (value >= startValue)
{
int next = value - (value - startValue) % increment + increment;
if (next <= endValue)
{
cronResult = new CronResult(next, 0);
}
}
}
else
{
cronResult = super.getNext(value, minValue, maxValue);
}
return cronResult;
}
@Override
public CronResult getPrevious(int value, int minValue, int maxValue)
{
CronResult cronResult = null;
if (getChronoField() == ChronoField.YEAR)
{
if (value > endValue)
{
value = endValue + 1;
}
if (value > startValue)
{
int mod = (value - startValue) % increment;
int prev = value;
if (mod == 0)
{
prev -= increment;
}
else
{
prev -= mod;
}
// (prev <= endValue) is always true, because we correct the value if it was greater then the
// endValue
cronResult = new CronResult(prev, 0);
}
}
else
{
cronResult = super.getPrevious(value, minValue, maxValue);
}
return cronResult;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy