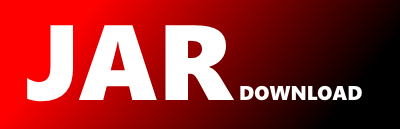
com.payu.auth.client.configuration.logging.AbstractLoggingAspect Maven / Gradle / Ivy
package com.payu.auth.client.configuration.logging;
import java.util.Objects;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.lang3.StringUtils;
import org.aspectj.lang.JoinPoint;
import org.aspectj.lang.ProceedingJoinPoint;
import org.slf4j.MDC;
import org.springframework.security.core.Authentication;
import org.springframework.security.core.context.SecurityContextHolder;
import org.springframework.security.oauth2.server.resource.authentication.JwtAuthenticationToken;
/**
* Mise en place des loggers automatiques au niveau des controllers, services, repositories,
* producers et consumers.
*/
@Slf4j
public abstract class AbstractLoggingAspect {
private static final String SYSTEM_USER = "-PAYU-";
private static final int ARGUMENT_TAILLE_LIMITE = 40;
/**
* Log information user.
*
* @param level le niveau de log afin de faire un décalage.
* @param joinPoint le point de jointure.
*/
protected void log(int level, JoinPoint joinPoint) {
MDC.put("USER_NAME", getConnectedUser());
StringBuilder logBuffer = new StringBuilder();
if (level == 0) {
logBuffer.append("[ DEBUT ] ");
} else {
logBuffer.append(StringUtils.repeat(" ", level));
}
logBuffer.append(joinPoint.getSignature().toShortString());
if (log.isDebugEnabled()) {
logBuffer.append(" - ").append(displayArgs(joinPoint.getArgs()));
}
log.info(logBuffer.toString());
}
/**
* Cette méthode permet de logguer la fin du traitement, pour un contrôleur ou un kafkaConsumer
* par exemple.
*
* @param joinPoint le point de jointure de l'aspect.
* @param startTime la date de démarrage du traitement sous forme de long.
* @param result le résultat de traitement afin de le logguer
*/
protected void logEnd(ProceedingJoinPoint joinPoint, long startTime, Object result) {
long executionTime = System.currentTimeMillis() - startTime;
StringBuilder endLog = new StringBuilder();
endLog
.append("[ FIN ] ")
.append(joinPoint.getSignature().toShortString())
.append(" en ")
.append(executionTime)
.append(" ms");
if (log.isDebugEnabled()) {
endLog.append(" - ").append(displayArgs(result));
}
log.info(endLog.toString());
}
/**
* Récupère l'utilisateur connecté de la session Spring Security.
*
* @return username connected user.
*/
protected String getConnectedUser() {
final Authentication authentication = SecurityContextHolder.getContext().getAuthentication();
if (authentication == null) {
return SYSTEM_USER;
}
if (!authentication.isAuthenticated()) {
return SYSTEM_USER;
}
if (authentication instanceof JwtAuthenticationToken jwtAuthenticationToken) {
return String.valueOf(jwtAuthenticationToken.getTokenAttributes().get("uid"));
} else {
return SYSTEM_USER;
}
}
/**
* Cette méthode permet d'afficher les arguments / retours des méthodes sous forme |REFERENCE| |un
* autre argument| |AUTRE CHOSE| Chaque argument est limité à 40 caractères.
*
* @param args la liste des arguments à transformer en string.
* @return le liste des arguments sous forme de string |REFERENCE| |un autre argument| |AUTRE
* CHOSE|
*/
protected String displayArgs(Object... args) {
return Stream.of(args)
.filter(Objects::nonNull)
.map(
a ->
StringUtils.wrap(StringUtils.abbreviate(a.toString(), ARGUMENT_TAILLE_LIMITE), "|"))
.collect(Collectors.joining(" "));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy