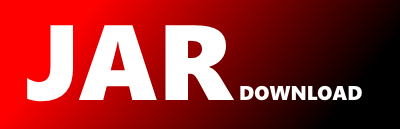
com.payu.auth.client.configuration.security.FirebaseJwtRolesConverter Maven / Gradle / Ivy
package com.payu.auth.client.configuration.security;
import com.payu.auth.client.enums.RoleType;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.stream.Collectors;
import org.springframework.core.convert.converter.Converter;
import org.springframework.security.authentication.AbstractAuthenticationToken;
import org.springframework.security.core.GrantedAuthority;
import org.springframework.security.core.authority.SimpleGrantedAuthority;
import org.springframework.security.oauth2.jwt.Jwt;
import org.springframework.security.oauth2.server.resource.authentication.JwtAuthenticationToken;
import org.springframework.stereotype.Component;
@Component
public class FirebaseJwtRolesConverter implements Converter {
/** Name of the claim containing roles. (Applicable to realm and resource level.) */
private static final String CLAIM_ROLES = "Roles";
/** Prefix used in combination with the resource (client) name for resource level roles. */
public static final String PREFIX_RESOURCE_ROLE = "ROLE_";
/**
* Extracts the realm and resource level roles from a JWT token distinguishing between them using
* prefixes.
*/
@Override
public AbstractAuthenticationToken convert(Jwt jwt) {
// Collection that will hold the extracted roles
Collection grantedAuthorities = new ArrayList<>();
// Realm roles
// Get the part of the access token that holds the roles assigned on realm level
Collection roles = jwt.getClaim(CLAIM_ROLES);
// Check if any roles are present
if (roles != null && !roles.isEmpty()) {
// Iterate of the roles and add them to the granted authorities
Collection realmRoles =
roles.stream()
.filter(
rle ->
Arrays.stream(RoleType.values())
.anyMatch(roleType -> roleType.name().equalsIgnoreCase(rle)))
.map(role -> new SimpleGrantedAuthority(PREFIX_RESOURCE_ROLE + role))
.collect(Collectors.toList());
grantedAuthorities.addAll(realmRoles);
} else {
// By default add User role
grantedAuthorities.add(
new SimpleGrantedAuthority(PREFIX_RESOURCE_ROLE + RoleType.USER.name()));
}
return new JwtAuthenticationToken(jwt, grantedAuthorities);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy