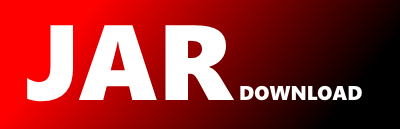
com.payu.auth.client.configuration.security.TokenInfo Maven / Gradle / Ivy
package com.payu.auth.client.configuration.security;
import com.payu.auth.client.enums.RoleType;
import com.payu.auth.client.enums.UserType;
import java.util.Arrays;
import java.util.Set;
import java.util.stream.Collectors;
import org.springframework.security.core.Authentication;
import org.springframework.security.core.context.SecurityContextHolder;
import org.springframework.stereotype.Component;
@Component
public class TokenInfo {
/**
* Get all info decoded in the token
*
* @return Authentication object contain information on the token
*/
public Authentication getTokenInfo() {
// Get the authentication object from the SecurityContextHolder
Authentication authentication = SecurityContextHolder.getContext().getAuthentication();
return (authentication != null && authentication.isAuthenticated()) ? authentication : null;
}
/**
* Get user uid from token
*
* @return User uid
*/
public String getUserIdFromToken() {
Authentication authentication = getTokenInfo();
return authentication != null ? authentication.getName() : null;
}
/**
* Get user type from token
*
* @return User type
*/
public UserType getUserTypeFromToken() {
Authentication authentication = getTokenInfo();
if (authentication != null) {
Set roles =
authentication.getAuthorities().stream()
.map(grantedAuthority -> grantedAuthority.getAuthority().replace("ROLE_", ""))
.collect(Collectors.toSet());
Set roleTypes =
Arrays.stream(RoleType.values())
.filter(
roleType ->
roles.stream().anyMatch(role -> roleType.name().equalsIgnoreCase(role)))
.collect(Collectors.toSet());
if (roleTypes.contains(RoleType.SUPER_ADMIN)) {
return UserType.SUPER_ADMIN;
} else if (roleTypes.contains(RoleType.ADMIN_PARTNER)) {
return UserType.ADMIN_PARTNER;
} else if (roleTypes.contains(RoleType.ADMIN)) {
return UserType.ADMIN;
} else {
return UserType.USER;
}
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy