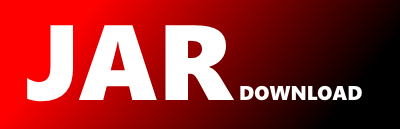
com.payu.common.client.handler.BadRequestErrorHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ms-common-client Show documentation
Show all versions of ms-common-client Show documentation
Librairie commune pour les modules MS Clients
package com.payu.common.client.handler;
import com.payu.common.client.error.ApiError;
import com.payu.common.client.exception.BadRequestException;
import com.payu.common.client.exception.IntermoduleError;
import java.util.HashMap;
import java.util.Map;
import java.util.Objects;
import lombok.extern.slf4j.Slf4j;
import org.slf4j.MDC;
import org.springframework.core.Ordered;
import org.springframework.core.annotation.Order;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.validation.FieldError;
import org.springframework.web.bind.MethodArgumentNotValidException;
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.method.annotation.MethodArgumentTypeMismatchException;
/** Handler des exceptions @{@link BadRequestException} */
@ControllerAdvice
@Slf4j
@Order(Ordered.HIGHEST_PRECEDENCE)
public class BadRequestErrorHandler extends AbstractErrorHandler {
/**
* @param exception exception traitant les ressources non trouvée en base
* @return une 404 enrichit avec l'erreur reformatée
*/
@ExceptionHandler({BadRequestException.class})
public ResponseEntity handleAll(Exception exception) {
log.warn("Validation error : {}", exception.getLocalizedMessage());
ApiError apiError =
ApiError.builder()
.status(HttpStatus.BAD_REQUEST)
.code(IntermoduleError.ERREUR_VALIDATION.getCode())
.message(
String.format(
"Technical error (correlationId: %s)", MDC.get(ApiError.TRACE_ID_Key)))
.detail(exception.getLocalizedMessage())
.correlationId((MDC.get(ApiError.TRACE_ID_Key)))
.build();
return new ResponseEntity<>(apiError, apiError.getStatus());
}
@ExceptionHandler(MethodArgumentNotValidException.class)
public ResponseEntity
© 2015 - 2024 Weber Informatics LLC | Privacy Policy