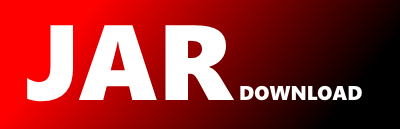
com.payu.common.client.handler.DefaultResponseErrorHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ms-common-client Show documentation
Show all versions of ms-common-client Show documentation
Librairie commune pour les modules MS Clients
package com.payu.common.client.handler;
import java.io.IOException;
import java.nio.charset.Charset;
import java.nio.charset.StandardCharsets;
import org.springframework.core.log.LogFormatUtils;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpStatus;
import org.springframework.http.MediaType;
import org.springframework.http.client.ClientHttpResponse;
import org.springframework.lang.Nullable;
import org.springframework.util.FileCopyUtils;
import org.springframework.util.ObjectUtils;
import org.springframework.web.client.HttpClientErrorException;
import org.springframework.web.client.HttpServerErrorException;
import org.springframework.web.client.ResponseErrorHandler;
import org.springframework.web.client.UnknownHttpStatusCodeException;
public class DefaultResponseErrorHandler implements ResponseErrorHandler {
public DefaultResponseErrorHandler() {
/* TODO document why this constructor is empty */
}
@Override
public boolean hasError(ClientHttpResponse response) throws IOException {
int rawStatusCode = response.getRawStatusCode();
HttpStatus statusCode = HttpStatus.resolve(rawStatusCode);
return statusCode != null ? this.hasError(statusCode) : this.hasError(rawStatusCode);
}
protected boolean hasError(HttpStatus statusCode) {
return statusCode.isError();
}
protected boolean hasError(int unknownStatusCode) {
HttpStatus.Series series = HttpStatus.Series.resolve(unknownStatusCode);
return series == HttpStatus.Series.CLIENT_ERROR || series == HttpStatus.Series.SERVER_ERROR;
}
@Override
public void handleError(ClientHttpResponse response) throws IOException {
HttpStatus statusCode = HttpStatus.resolve(response.getRawStatusCode());
if (statusCode == null) {
byte[] body = this.getResponseBody(response);
String message =
this.getErrorMessage(
response.getRawStatusCode(),
response.getStatusText(),
body,
this.getCharset(response));
throw new UnknownHttpStatusCodeException(
message,
response.getRawStatusCode(),
response.getStatusText(),
response.getHeaders(),
body,
this.getCharset(response));
} else {
this.handleError(response, statusCode);
}
}
protected void handleError(ClientHttpResponse response, HttpStatus statusCode)
throws IOException {
String statusText = response.getStatusText();
HttpHeaders headers = response.getHeaders();
byte[] body = this.getResponseBody(response);
Charset charset = this.getCharset(response);
String message = this.getErrorMessage(statusCode.value(), statusText, body, charset);
switch (statusCode.series()) {
case CLIENT_ERROR -> throw HttpClientErrorException.create(
message, statusCode, statusText, headers, body, charset);
case SERVER_ERROR -> throw HttpServerErrorException.create(
message, statusCode, statusText, headers, body, charset);
default -> throw new UnknownHttpStatusCodeException(
message, statusCode.value(), statusText, headers, body, charset);
}
}
private String getErrorMessage(
int rawStatusCode,
String statusText,
@Nullable byte[] responseBody,
@Nullable Charset charset) {
String preface = rawStatusCode + " " + statusText + " " + ": ";
if (ObjectUtils.isEmpty(responseBody)) {
return preface + "[no body]";
} else {
charset = charset != null ? charset : StandardCharsets.UTF_8;
String bodyText = new String(responseBody, charset);
bodyText = LogFormatUtils.formatValue(bodyText, -1, true);
return preface + bodyText;
}
}
protected byte[] getResponseBody(ClientHttpResponse response) {
try {
return FileCopyUtils.copyToByteArray(response.getBody());
} catch (IOException var3) {
return new byte[0];
}
}
@Nullable
protected Charset getCharset(ClientHttpResponse response) {
HttpHeaders headers = response.getHeaders();
MediaType contentType = headers.getContentType();
return contentType != null ? contentType.getCharset() : null;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy