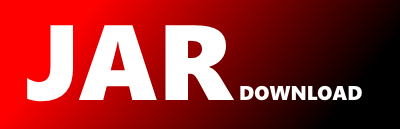
com.payu.common.client.handler.IntermoduleRestTemplateResponseErrorHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ms-common-client Show documentation
Show all versions of ms-common-client Show documentation
Librairie commune pour les modules MS Clients
package com.payu.common.client.handler;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.payu.common.client.error.ApiClientError;
import com.payu.common.client.exception.IntermoduleException;
import java.io.IOException;
import java.nio.charset.StandardCharsets;
import java.util.Map;
import lombok.NoArgsConstructor;
import org.springframework.http.HttpStatus;
import org.springframework.http.client.ClientHttpResponse;
import org.springframework.util.ObjectUtils;
import org.springframework.web.client.UnknownHttpStatusCodeException;
/**
* Handler permettant de generer les erreurs levees aux niveau des clients restTemplate des modules
*/
@NoArgsConstructor
public class IntermoduleRestTemplateResponseErrorHandler extends DefaultResponseErrorHandler {
private static final int BODY_MAX_CHARS = 200;
@Override
public boolean hasError(ClientHttpResponse response) throws IOException {
HttpStatus statusCode = (HttpStatus) response.getStatusCode();
return (statusCode.is5xxServerError() || statusCode.is4xxClientError());
}
@Override
public void handleError(ClientHttpResponse response) throws IOException {
HttpStatus statusCode = HttpStatus.resolve(response.getRawStatusCode());
String errorMessage;
if (statusCode == null) {
throw new UnknownHttpStatusCodeException(
"Status HTTP inconnu",
response.getRawStatusCode(),
response.getStatusText(),
response.getHeaders(),
getResponseBody(response),
getCharset(response));
}
ApiClientError apiClientError =
ApiClientError.builder().message(getApiErrorMessage(response)).status(statusCode).build();
try {
errorMessage = getErrorMessage(response.getStatusText(), apiClientError);
} catch (Exception exception) {
errorMessage = apiClientError.getMessage();
}
throw new IntermoduleException(errorMessage, apiClientError);
}
protected String getApiErrorMessage(ClientHttpResponse response) throws IOException {
if (ObjectUtils.isEmpty(response.getBody())) {
return "[no body]";
}
return new String(getResponseBody(response), StandardCharsets.UTF_8);
}
/**
* @param statusText status text de la response
* @param apiClientError Objet apiClientEror
* @return le message d'erreur
* @throws JsonProcessingException
*/
protected String getErrorMessage(String statusText, ApiClientError apiClientError)
throws JsonProcessingException {
Map mapMessageErreur =
new ObjectMapper().readValue(apiClientError.getMessage(), Map.class);
return statusText
.concat(" - CorrelationId : ")
.concat(mapMessageErreur.get("correlationId"))
.concat("- ErrorMessage : ")
.concat(mapMessageErreur.get("message"));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy