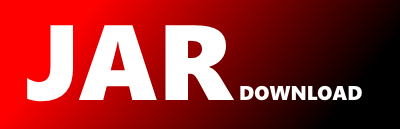
org.cattleframework.cloud.discovery.DiscoveryInitialize Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cattle-cloud-discovery-common Show documentation
Show all versions of cattle-cloud-discovery-common Show documentation
Cattle framework cloud discovery common component pom
/*
* Copyright (C) 2018 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.cattleframework.cloud.discovery;
import java.time.Duration;
import java.util.List;
import java.util.stream.Collectors;
import org.apache.commons.collections4.CollectionUtils;
import org.apache.commons.lang3.StringUtils;
import org.cattleframework.aop.event.ContextInitializeEvent;
import org.cattleframework.aop.processor.SortedBeanPostProcessor;
import org.cattleframework.aop.utils.ServiceLoaderUtils;
import org.cattleframework.cloud.discovery.processor.DiscoveryPropertiesProcessor;
import org.cattleframework.cloud.discovery.reflect.DiscoveryClientProxy;
import org.springframework.beans.BeansException;
import org.springframework.beans.factory.config.ConfigurableListableBeanFactory;
import org.springframework.cloud.client.discovery.DiscoveryClient;
import org.springframework.cloud.loadbalancer.cache.LoadBalancerCacheProperties;
import org.springframework.core.Ordered;
import org.springframework.core.env.Environment;
/**
* 服务发现初始化
*
* @author orange
*
*/
public class DiscoveryInitialize implements ContextInitializeEvent {
@Override
public void execute(ConfigurableListableBeanFactory beanFactory, Environment environment) throws Throwable {
List discoveryPropertiesProcessors = ServiceLoaderUtils
.getService(DiscoveryPropertiesProcessor.class);
String processorName = StringUtils.uncapitalize(getClass().getSimpleName()) + "Processor";
beanFactory.registerSingleton(processorName, new SortedBeanPostProcessor() {
@Override
public Object postProcessAfterInitialization(Object bean, String beanName) throws BeansException {
if (bean instanceof DiscoveryClient) {
DiscoveryClient target = (DiscoveryClient) bean;
return DiscoveryClientProxy.getDiscoveryClientProxy(beanFactory, target);
} else if (bean instanceof LoadBalancerCacheProperties) {
LoadBalancerCacheProperties loadBalancerCacheProperties = (LoadBalancerCacheProperties) bean;
LoadBalancerProperties loadBalancerProperties = beanFactory.getBean(LoadBalancerProperties.class);
if (loadBalancerProperties.isCache()) {
loadBalancerCacheProperties.setTtl(Duration.ofSeconds(loadBalancerProperties.getTimeToLive()));
loadBalancerCacheProperties.setCapacity(loadBalancerProperties.getCapacity());
}
return loadBalancerCacheProperties;
} else {
if (CollectionUtils.isNotEmpty(discoveryPropertiesProcessors)) {
List discoveryPropertiesProcessorLst = discoveryPropertiesProcessors
.stream().filter(p -> p.needProcess(bean.getClass())).collect(Collectors.toList());
if (CollectionUtils.isNotEmpty(discoveryPropertiesProcessorLst)) {
DiscoveryInfoProperties discoveryInfoProperties = beanFactory
.getBean(DiscoveryInfoProperties.class);
discoveryPropertiesProcessorLst.forEach(
discoveryPropertiesProcessor -> discoveryPropertiesProcessor.process(beanFactory,
environment, bean, discoveryInfoProperties, beanFactory
.getBean(discoveryPropertiesProcessor.getSourcePropertiesClass())));
}
}
return bean;
}
}
@Override
public int getOrder() {
return Ordered.HIGHEST_PRECEDENCE;
}
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy