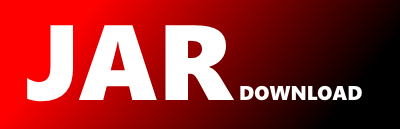
org.cattleframework.db.datasource.reflect.invocation.ConnectionInvocationHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cattle-db-datasource Show documentation
Show all versions of cattle-db-datasource Show documentation
Cattle framework db data source component pom
The newest version!
/*
* Copyright (C) 2018 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.cattleframework.db.datasource.reflect.invocation;
import java.lang.reflect.Method;
import java.sql.Blob;
import java.sql.Clob;
import java.sql.Connection;
import java.sql.NClob;
import java.sql.PreparedStatement;
import java.sql.Statement;
import org.cattleframework.db.datasource.DataSourceConstants;
import org.cattleframework.utils.reflect.ReflectUtils;
import org.checkerframework.checker.nullness.qual.Nullable;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.google.common.reflect.AbstractInvocationHandler;
import com.google.common.reflect.Reflection;
/**
* 数据库连接调用处理
*
* @author orange
*
*/
public class ConnectionInvocationHandler extends AbstractInvocationHandler {
private final Logger logger = LoggerFactory.getLogger(getClass());
private static final String METHOD_NAME_GET_TARGET_CONNECTION = "getTargetConnection";
private static final String METHOD_NAME_PREPARE_STATEMENT = "prepareStatement";
private static final String METHOD_NAME_CREATE_STATEMENT = "createStatement";
private static final String METHOD_NAME_PREPARE_CALL = "prepareCall";
private static final String METHOD_NAME_CREATE_BLOB = "createBlob";
private static final String METHOD_NAME_CREATE_CLOB = "createClob";
private static final String METHOD_NAME_CREATE_NCLOB = "createNClob";
private static final String METHOD_NAME_CLOSE = "close";
private final Connection target;
private boolean logDebug = false;
private boolean logInfo = false;
public ConnectionInvocationHandler(Connection target) {
this.target = target;
if (logger.isDebugEnabled()) {
logDebug = true;
} else if (logger.isInfoEnabled()) {
logInfo = true;
}
}
private void log(String format, Object... arguments) {
if (logDebug) {
logger.debug(format, arguments);
} else if (logInfo) {
logger.info(format, arguments);
}
}
@Override
protected Object handleInvocation(Object proxy, Method method, @Nullable Object[] args) throws Throwable {
String methodName = method.getName();
if (DataSourceConstants.METHOD_NAME_UNWRAP.equals(methodName)) {
if (((Class>) args[0]).isInstance(proxy)) {
return proxy;
}
} else if (DataSourceConstants.METHOD_NAME_IS_WRAPPER_FOR.equals(methodName)) {
if (((Class>) args[0]).isInstance(proxy)) {
return true;
}
} else if (METHOD_NAME_GET_TARGET_CONNECTION.equals(methodName)) {
return target;
} else if (METHOD_NAME_PREPARE_STATEMENT.equals(methodName) || METHOD_NAME_PREPARE_CALL.equals(methodName)) {
return prepareStatement(method, args);
} else if (METHOD_NAME_CREATE_STATEMENT.equals(methodName)) {
return createStatement(method, args);
} else if (METHOD_NAME_CREATE_BLOB.equals(methodName)) {
return createBlob(method, args);
} else if (METHOD_NAME_CREATE_CLOB.equals(methodName)) {
return createClob(method, args);
} else if (METHOD_NAME_CREATE_NCLOB.equals(methodName)) {
return createNclob(method, args);
} else if (METHOD_NAME_CLOSE.equals(methodName)) {
return close(method, args);
}
return ReflectUtils.invokeObjectMethod(method, target, args);
}
private NClob createNclob(Method method, Object[] args) throws Throwable {
long startTime = System.currentTimeMillis();
NClob nclob = (NClob) ReflectUtils.invokeObjectMethod(method, target, args);
long endTime = System.currentTimeMillis();
log("创建NClob,执行时间:{}毫秒", (endTime - startTime));
return nclob;
}
private Clob createClob(Method method, Object[] args) throws Throwable {
long startTime = System.currentTimeMillis();
Clob clob = (Clob) ReflectUtils.invokeObjectMethod(method, target, args);
long endTime = System.currentTimeMillis();
log("创建Clob,执行时间:{}毫秒", (endTime - startTime));
return clob;
}
private Blob createBlob(Method method, Object[] args) throws Throwable {
long startTime = System.currentTimeMillis();
Blob blob = (Blob) ReflectUtils.invokeObjectMethod(method, target, args);
long endTime = System.currentTimeMillis();
log("创建Blob,执行时间:{}毫秒", (endTime - startTime));
return blob;
}
private Statement createStatement(Method method, Object[] args) throws Throwable {
Statement statement = (Statement) ReflectUtils.invokeObjectMethod(method, target, args);
return Reflection.newProxy(Statement.class, new StatementInvocationHandler(statement));
}
private PreparedStatement prepareStatement(Method method, Object[] args) throws Throwable {
String sql = (String) args[0];
PreparedStatement ps = (PreparedStatement) ReflectUtils.invokeObjectMethod(method, target, args);
return Reflection.newProxy(PreparedStatement.class, new PreparedStatementInvocationHandler(ps, sql));
}
private Object close(Method method, Object[] args) throws Throwable {
long startTime = System.currentTimeMillis();
Object result = ReflectUtils.invokeObjectMethod(method, target, args);
long endTime = System.currentTimeMillis();
log("关闭数据库连接,执行时间:{}毫秒", (endTime - startTime));
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy