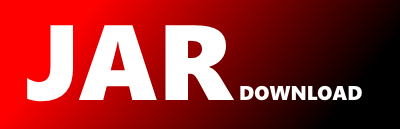
org.cattleframework.db.datasource.utils.DataSourceUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cattle-db-datasource Show documentation
Show all versions of cattle-db-datasource Show documentation
Cattle framework db data source component pom
The newest version!
/*
* Copyright (C) 2018 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.cattleframework.db.datasource.utils;
import java.lang.reflect.Method;
import java.lang.reflect.Parameter;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Vector;
import javax.sql.DataSource;
import org.apache.commons.lang3.StringUtils;
import org.cattleframework.aop.context.SpringContext;
import org.cattleframework.aop.utils.SimpleReflectUtils;
import org.cattleframework.db.datasource.druid.DruidDataSourceWrapper;
import org.cattleframework.db.datasource.security.DataSourceSecurityHandler;
import org.cattleframework.db.datasource.security.DataSourceSecurityInfo;
import org.cattleframework.exception.CattleException;
import org.cattleframework.exception.ExceptionWrapUtils;
import org.cattleframework.utils.auxiliary.DataConvertUtils;
import org.cattleframework.utils.reflect.ReflectUtils;
import com.alibaba.druid.filter.Filter;
import com.zaxxer.hikari.HikariDataSource;
/**
* 数据源工具
*
* @author orange
*
*/
public final class DataSourceUtils {
private static final String DRUID_CLASS_NAME = "org.cattleframework.db.datasource.druid.DruidDataSourceWrapper";
private static final String HIKARI_CLASS_NAME = "com.zaxxer.hikari.HikariDataSource";
private DataSourceUtils() {
}
private static Class> getDataSourceClass() {
Class> dataSourceClass = org.cattleframework.utils.reflect.ClassUtils.getClass(DRUID_CLASS_NAME, true);
if (dataSourceClass == null) {
dataSourceClass = org.cattleframework.utils.reflect.ClassUtils.getClass(HIKARI_CLASS_NAME, true);
}
if (dataSourceClass == null) {
throw new CattleException("没有找到数据源连接池");
}
return dataSourceClass;
}
public static DataSource getDataSource(String name, String driverClassName, String jdbcUrl, String username,
String password, String securityHandlerClassName, Map props) {
if (StringUtils.isBlank(jdbcUrl)) {
throw new CattleException((StringUtils.isNotBlank(name) ? "名称'" + name + "'的" : "") + "数据源JDBC URL为空");
}
Class> dataSourceClass = getDataSourceClass();
DataSource dataSource = (DataSource) SimpleReflectUtils.instance(dataSourceClass);
if (props != null) {
try {
setDataSource(dataSource, props);
} catch (Throwable e) {
throw ExceptionWrapUtils.wrap(e);
}
}
if (StringUtils.isNotBlank(securityHandlerClassName)) {
Class> securityHandlerClass = org.cattleframework.utils.reflect.ClassUtils
.getClass(securityHandlerClassName);
DataSourceSecurityHandler dataSourceSecurityHandler = (DataSourceSecurityHandler) SimpleReflectUtils
.instance(securityHandlerClass);
DataSourceSecurityInfo dataSourceSecurityInfo = dataSourceSecurityHandler.getSecurityInfo();
if (dataSourceSecurityInfo == null) {
throw new CattleException((StringUtils.isNotBlank(name) ? "名称'" + name + "'的" : "") + "数据源安全信息为空");
}
username = dataSourceSecurityInfo.getUsername();
password = dataSourceSecurityInfo.getPassword();
}
String className = dataSourceClass.getName();
if (DRUID_CLASS_NAME.equals(className)) {
((DruidDataSourceWrapper) dataSource).setDriverClassName(driverClassName);
((DruidDataSourceWrapper) dataSource).setUrl(jdbcUrl);
((DruidDataSourceWrapper) dataSource).setUsername(username);
((DruidDataSourceWrapper) dataSource).setPassword(password);
String[] beanNames = SpringContext.get().getBeanFactory().getBeanNamesForType(Filter.class);
List filters = new Vector();
for (int i = 0; i < beanNames.length; i++) {
filters.add((Filter) SpringContext.get().getBeanFactory().getBean(beanNames[i]));
}
((DruidDataSourceWrapper) dataSource).autoAddFilters(filters);
} else if (HIKARI_CLASS_NAME.equals(className)) {
((HikariDataSource) dataSource).setDriverClassName(driverClassName);
((HikariDataSource) dataSource).setJdbcUrl(jdbcUrl);
((HikariDataSource) dataSource).setUsername(username);
((HikariDataSource) dataSource).setPassword(password);
} else {
throw new CattleException((StringUtils.isNotBlank(name) ? "名称'" + name + "'的" : "") + "未知数据源连接池类:"
+ dataSourceClass.getName());
}
return dataSource;
}
private static void setDataSource(DataSource dataSource, Map props) throws Throwable {
Iterator iterator = props.keySet().iterator();
while (iterator.hasNext()) {
String key = iterator.next();
Object value = props.get(key);
String name = getKey(key);
Method method = ReflectUtils.findSetMethod(dataSource.getClass(), name);
if (method != null) {
Parameter parameter = method.getParameters()[0];
ReflectUtils.invokeObjectMethod(method, dataSource,
DataConvertUtils.convertValue(value, parameter.getType()));
}
}
}
private static String getKey(String key) {
StringBuffer sb = new StringBuffer();
int len = key.length();
for (int i = 0; i < len; i++) {
String var = key.substring(i, i + 1);
if ("-".equals(var)) {
if (i + 1 < len) {
var = key.substring(i + 1, i + 2).toUpperCase();
sb.append(var);
i++;
} else {
sb.append(var);
}
} else {
sb.append(var);
}
}
return sb.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy