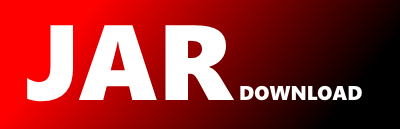
org.cattleframework.utils.auxiliary.Dom4jUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cattle-utils Show documentation
Show all versions of cattle-utils Show documentation
Cattle framework utils component pom
/*
* Copyright (C) 2018 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.cattleframework.utils.auxiliary;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.StringReader;
import java.net.URL;
import java.util.StringTokenizer;
import org.cattleframework.exception.ExceptionWrapUtils;
import org.dom4j.Document;
import org.dom4j.DocumentException;
import org.dom4j.Element;
import org.dom4j.io.OutputFormat;
import org.dom4j.io.SAXReader;
import org.dom4j.io.XMLWriter;
import org.xml.sax.InputSource;
import org.xml.sax.SAXException;
/**
* Dom4j工具
*
* @author orange
*
*/
public final class Dom4jUtils {
private static final String XML_MARK = "");
String sub = xml.substring(0, end);
StringTokenizer tokens = new StringTokenizer(sub, " =\"\'");
while (tokens.hasMoreTokens()) {
String token = tokens.nextToken();
if ("encoding".equals(token)) {
if (tokens.hasMoreTokens()) {
result = tokens.nextToken();
}
break;
}
}
}
return result;
}
/**
* 根据Document对象生成不带回车的Xml字符串
*
* @param document document对象
* @param enc 字符集
* @return 不带回车的Xml字符串
*/
public static String asXmlWithoutPretty(Document document, String enc) {
return asXml(document, enc, false);
}
/**
* 根据Document对象生成带回车的Xml字符串
*
* @param document document对象
* @param enc 字符集
* @return 带回车的Xml字符串
*/
public static String asXmlWithPretty(Document document, String enc) {
return asXml(document, enc, true);
}
/**
* 根据Document对象生成Xml字符串
*
* @param document document对象
* @param enc 字符集
* @param isPretty 是否带有漂亮的格式
* @return Xml字符串
*/
private static String asXml(Document document, String enc, boolean isPretty) {
XMLWriter writer = null;
ByteArrayOutputStream bout = null;
try {
String xml = "";
// 美化格式
OutputFormat format = null;
if (isPretty) {
format = OutputFormat.createPrettyPrint();
} else {
format = OutputFormat.createCompactFormat();
}
format.setEncoding(enc);
format.setTrimText(false);
format.setExpandEmptyElements(false);
format.setNewLineAfterDeclaration(false);
bout = new ByteArrayOutputStream();
writer = new XMLWriter(bout, format);
if (document != null) {
writer.write(document);
xml = bout.toString(enc);
}
return xml;
} catch (IOException e) {
throw ExceptionWrapUtils.wrap(e);
} finally {
try {
if (writer != null) {
writer.close();
}
if (bout != null) {
bout.close();
}
} catch (IOException e) {
}
}
}
/**
* 根据Element对象生成不带回车的Xml字符串
*
* @param element element对象
* @param enc 字符集
* @return 不带回车的Xml字符串
*/
public static String asXmlWithoutPretty(Element element, String enc) {
return asXml(element, enc, false);
}
/**
* 根据Element对象生成带回车的Xml字符串
*
* @param element element对象
* @param enc 字符集
* @return 带回车的Xml字符串
*/
public static String asXmlWithPretty(Element element, String enc) {
return asXml(element, enc, true);
}
/**
* 根据Element对象生成Xml字符串
*
* @param element element对象
* @param enc 字符集
* @param isPretty 是否带有漂亮的格式
* @return Xml字符串
*/
private static String asXml(Element element, String enc, boolean isPretty) {
XMLWriter writer = null;
try (ByteArrayOutputStream baos = new ByteArrayOutputStream()) {
String xml = "";
// 美化格式
OutputFormat format = null;
if (isPretty) {
format = OutputFormat.createPrettyPrint();
} else {
format = OutputFormat.createCompactFormat();
}
format.setEncoding(enc);
format.setTrimText(false);
format.setExpandEmptyElements(false);
format.setNewLineAfterDeclaration(false);
writer = new XMLWriter(baos, format);
if (element != null) {
writer.write(element);
xml = baos.toString(enc);
}
return xml;
} catch (IOException e) {
throw ExceptionWrapUtils.wrap(e);
} finally {
try {
if (writer != null) {
writer.close();
}
} catch (IOException e) {
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy